No, this is not about my patent pending idea of a sheet splitter that turns duplex documents into simplex documents… This post is about a problem that comes up every now and then: When you scan a book or a magazine, chances are that you end up with two physical pages on your scanned image, and your document looks something like this:
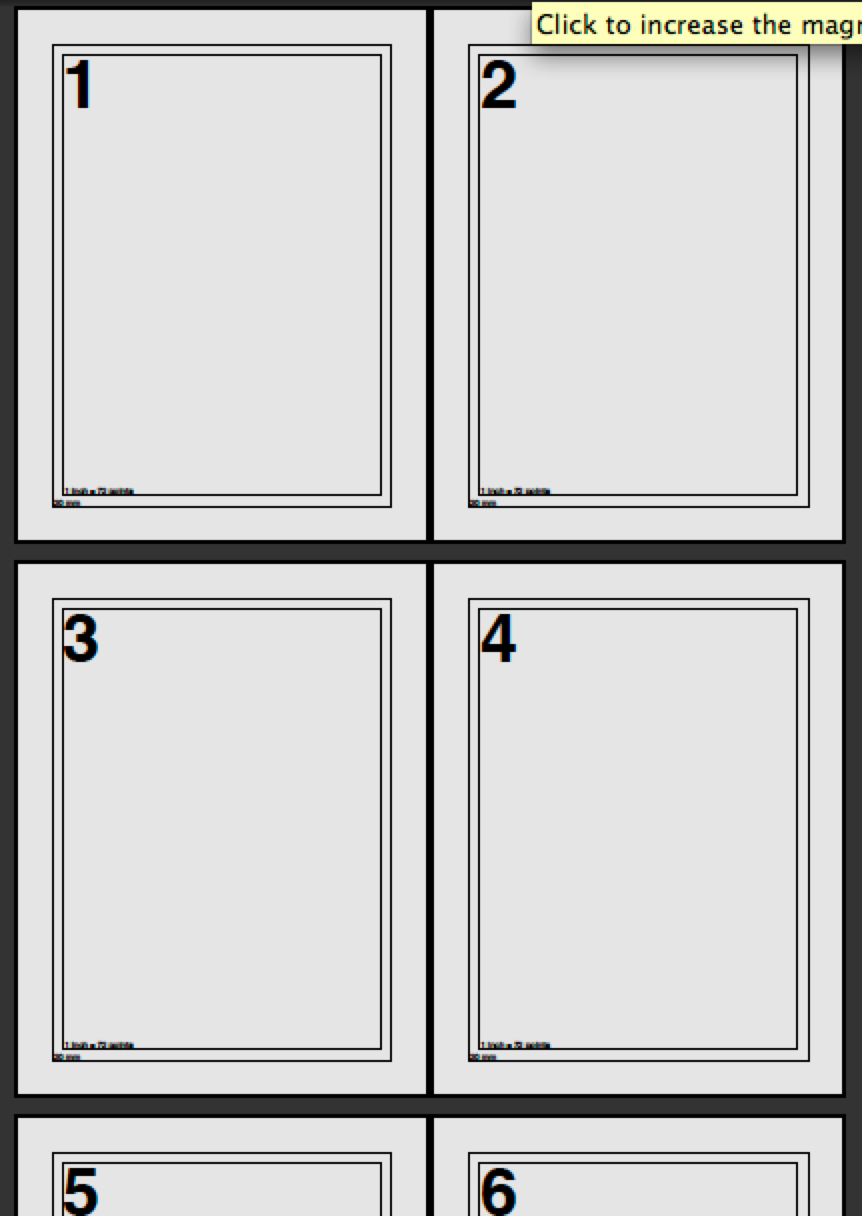
Pages one and two are on the same scan, three and four are, and five and six and so on.
How can we split such a combined page into it’s two parts?
There are of course different solutions to this problem, some more complicated than others, some producing better results than others.
The most straight forward approach would be to write an Acrobat plug-in or a standalone application (e.g. using the iText library) that takes the source page, determines what needs to be copied to the new page that should represent the left half of the original page, and then just copy those page elements. With a scanned source document, this would potentially mean that the scanned image needs to be cropped and placed on the target page. Sounds complicated, and it is complicated. Is there an easier way to accomplish the same results?
If we have access the Acrobat, we can use JavaScript to mimic the behavior of the just described application. With Acrobat’s JavaScript, we do of course not have access to the page content elements, so we need to find a different way to end up with the same results.
JavaScript gives us access to the crop box of a PDF page (if you don’t know what that is, read up on page boxes in the PDF Reference), this means we can find out how big a page is, but also set the crop box to configure which part of the page should be displayed in the viewer, or should get printed.
So, if our combined page is 11×17″, and we want to extract two 8 1/2×11″ pages, we first need to “select” the left half of the page, and then the right half of the same page.
Here is the script that will do just that:
SplitPages.js
Copy that file to the Acrobat JavaScript directory – for Acrobat 9 on a Windows machine that would be
c:\Program Files\Adobe\Acrobat 9.0\Acrobat\JavaScript
Now let’s take a look at the different parts of the script:
ProcessDocument = app.trustedFunction(function()
{
// create a new document
app.beginPriv();
var newDoc = app.newDoc();
app.endPriv();
This snippet shows that we are declaring a trusted function. This is necessary because we need to execute the app.newDoc() method, which requires (since Acrobat 7) a privileged context. The first thing we do in this script is to create that new document – the call is wrapped with the beginPriv() and endPriv() calls. When creating a new document in JavaScript, the document is no only created, Acrobat will also add a page to that document. We don’t need that page, but every PDF document that is getting displayed in Acrobat, needs at least one PDF page. We will deal with that extra page later.
var i = 0; while (i < this.numPages) { newDoc.insertPages( { nPage: newDoc.numPages-1, cPath: this.path, nStart: i }); newDoc.insertPages( { nPage: newDoc.numPages-1, cPath: this.path, nStart: i }); // we did this twice so that we can then split each copy of the page into a left // and right half. i++; }
In these few lines we copy every page from the source document (this.path, which is the path to the active document) to our newly created document – and we are doing that twice. This is necessary because we need to crop out the left half of the page for the first page, and the right half of the page for the second page. After this loop, we will have twice as many pages in our new document than we have in our source document.
if (newDoc.numPages > 1) { newDoc.deletePages(0); // this gets rid of the page that was created with the newDoc call. }
Now that we have all the pages in our new document, we no longer need the blank page we got when we created the document. So, we delete it.
// at this point we have a documnent with every page from the source document
// copied twice
for (i=0; i<newDoc.numPages; i++) { // determine the crop box of the page var cropRect = newDoc.getPageBox("Crop", i); var halfWidth = (cropRect[2]-cropRect[0])/2;
We loop over all pages in our new document (that is twice the number of pages in the original document). And for every page we get the crop box. We also calculate a value that we will need later: The half of the width of that page. That is the location where the page will get split.
var cropLeft = new Array();
cropLeft[0] = cropRect[0];
cropLeft[1] = cropRect[1];
cropLeft[2] = cropRect[0] + halfWidth;
cropLeft[3] = cropRect[3];
The previous few lines create a new Array. A page box is represented as an array of four values. We can now assign the partially modified values to the new page box. As you can see, three of the four values just are copies of the original crop box. Array element 2 however gets modified. It is the original X value for the lower left point of the crop box, and we add half of our page width to that number. This means, the new right edge of the modified page box is now at the halfway point between the two sides.
var cropRight = new Array();
cropRight[0] = cropRect[2] - halfWidth;
cropRight[1] = cropRect[1];
cropRight[2] = cropRect[2];
cropRight[3] = cropRect[3];
We do something similar for the new crop box for the right side of the page.
if (i%2 == 0)
{
newDoc.setPageBoxes( {
cBox: "Crop",
nStart: i,
rBox: cropLeft
});
}
else
{
newDoc.setPageBoxes( {
cBox: "Crop",
nStart: i,
rBox: cropRight
});
}
}
}
)
This is a bit tricky… Acrobat starts to count pages with page 0. The first page in the document is on the left half of our first sheet. All of a sudden, our first page is actually an even page number (0), and not like in “normal” books an odd number (1). This means that for all even numbers we need to crop the left half, and for all odd numbers we need to crop the right half.
We test for “evenness” by performing the modulo operation on our page number. If the result is 0, we know we have an even number, so we can use the left side crop box. If the operation returns 1, we are dealing with an odd page number, and we will use the ride side crop box.
The new crop box gets applied with the doc.setPageBoxes() method.
// add the menu item
app.addMenuItem({
cName: "splitPagesJS", // this is the internal name used for this menu item
cUser: "Split Pages", // this is the label that is used to display the menu item
cParent: "Document", // this is the parent menu. The file menu would use "File"
cExec: "ProcessDocument()", // this is the JavaScript code to execute when this menu item is selected
cEnable: "event.rc = (event.target != null);", // when should this menu item be active?
nPos: 0
});
Almost done… We’ve implemented the functionality to split the pages, now we just need a mechanism to actually start our little program. I’ve chosen to create a menu item under the “Document” menu. That menu item is only available (not grayed out), if we have an active document.
Disclaimer: Of course, there is no such thing as a sheet splitter, and therefore there is no patent application. It’s a joke, so don’t sue me if you actually have a patent on a sheet splitter š
Brilliant, Thank you very much. Now I only have to learn how to use it.
Wow, thanks! Works great! (I had to adapt the script to Acrobat 6).
Hi, thats amazing exactly what I was searching for. Based on this neat little snipplet I added a reorder java script which resequentialises booklet pages, i.e. booklet or broshures scanned doublesided two pages on a side. If you apply document/reorder after document/split, the pdf pages are in sequentiel order again. Here is the code:
ReorderDocument = app.trustedFunction(function()
{
// create a new document
app.beginPriv();
var newDoc = app.newDoc();
app.endPriv();
// reorder pages in booklet order into new document in sequential order
var i = 0;
var n = this.numPages;
for (var i = 1; i <= n / 4; i++) { newDoc.insertPages( { nPage: newDoc.numPages-1, cPath: this.path, nStart: i * 4 - 3, nEnd: i * 4 - 2 }); } newDoc.insertPages( { nPage: newDoc.numPages - 1, cPath: this.path, nStart: n - 1 }); for (var i = 1; i <= n / 4 - 1; i++) { newDoc.insertPages( { nPage: newDoc.numPages - 1, cPath: this.path, nStart: n - i * 4 }); newDoc.insertPages( { nPage: newDoc.numPages - 1, cPath: this.path, nStart: n - i * 4 - 1 }); } newDoc.insertPages( { nPage: newDoc.numPages - 1, cPath: this.path, nStart: 0 }); if (newDoc.numPages > 1)
{
newDoc.deletePages(0); // this gets rid of the page that was created with the newDoc call.
}
})
// add the menu item
app.addMenuItem({
cName: “reorderPagesJS”, // this is the internal name used for this menu item
cUser: “Reorder Pages”, // this is the label that is used to display the menu item
cParent: “Document”, // this is the parent menu. The file menu would use “File”
cExec: “ReorderDocument()”, // this is the JavaScript code to execute when this menu item is selected
cEnable: “event.rc = (event.target != null);”, // when should this menu item be active?
nPos: 1
});
GREAT!!!! Exactly what I was looking for! Great how easy pages can be splitted!
@Dirk:
I tried your js as well, but I always receive an error message:
“syntax error
14:Folder-Level:App:2_sortpages.js”
I use A9Pro Extended and copied the source 1:1. Can you give me a hint what to change?
Thanks
Fritz
The script is wonderful. Thank you.
Remember to change the setting to allow execution of javascript from the menu in Preferences
Thanks for the reply. Yes, you need to make sure that the menu setting is enabled (which is the problem that Fritz ran into earlier).
If you are still around.
The scripts are awesome. Exactly what we need.
I would like to use the re-order script as well.
I keep getting the same error as Dirk Rother.
Ć¢ā¬Åsyntax error
14:Folder-Level:reorderPages.jsĆ¢ā¬Ā.
I’ve enabled “Enable menu items JavaScript exectuion privileges” in the Preference menu. If you have any further suggestions, or maybe I’m looking in the incorrect spot.
Using Adobe 9 Standard.
Thanks guys, great stuff.
There is a space between “<" and "=" on line `14 in Dirk's script. when you remove that, it should work.
Thanks Kirk.
Using what Kirk sent, I removed that space from Dirk’s script.
Next error was on Line 63. I took the part from the original script that inserts the buttons, paste into this one, and just changed the names accordingly.
Works great now.
I could not find the difference. But when I loaded them both into a javascript editor I downloaded, it identified them as different colors (the last portion). Figured something was coming up incorrectly.
Thanks again guys, works great now.
ReorderDocument = app.trustedFunction(function()
{
// create a new document
app.beginPriv();
var newDoc = app.newDoc();
app.endPriv();
// reorder pages in booklet order into new document in sequential order
var i = 0;
var n = this.numPages;
for (var i = 1; i <= n / 4; i++)
{
newDoc.insertPages( {
nPage: newDoc.numPages-1,
cPath: this.path,
nStart: i * 4 – 3,
nEnd: i * 4 – 2
});
}
newDoc.insertPages( {
nPage: newDoc.numPages – 1,
cPath: this.path,
nStart: n – 1
});
for (var i = 1; i 1)
{
newDoc.deletePages(0); // this gets rid of the page that was created with the newDoc call.
}
})
// add the menu item
app.addMenuItem({
cName: “reorderPagesJS”, // this is the internal name used for this menu item
cUser: “Reorder Pages”, // this is the label that is used to display the menu item
cParent: “Document”, // this is the parent menu. The file menu would use “File”
cExec: “ReorderDocument()”, // this is the JavaScript code to execute when this menu item is selected
cEnable: “event.rc = (event.target != null);”, // when should this menu item be active?
nPos: 1
});
This is wonderful script. IĀ“m struggling (and few million other InDesign CS5 users) with inDesign CS5Ā“s new interactive PDF. It makes all PDF by spreads. Cover page (and usually last page too) is single page and rest of the pages comes as pairs… so page 1 is single page, but pages 2 and 3 are in PDFĀ“s page 2, pages 4 and 5 in PDFĀ“s page 3 and so on… so you script is very usefull. ThereĀ“s few things still that I would like to ask.
1) would it be hard to change it so that it didn“t split first page, because it“s practically always single page. Last page is also very often single page.
2) Now it seems to leave some interactive objects “floating” outside of page after splittind. For instance if I have a button in page 3 (page 2 in original PDF). After splitting, that button is exactly right place in page 3, but itĀ“s also in page 2, but outside of the page, in a grey area.
IĀ“d like to ecommend this script anyway to InDesign users… really great job…
Petteri, try this file instead:
http://www.khk.net/wordpress/wp-content/uploads/2010/09/splitpages.js
It does not process the first page, and only processes the last page when itās size is twice that of the first page. It also hides the form fields that show up floating outside of the crop box.
You sir, are my hero. This script rocks my face off.
Fantastic work!
Pingback: Acrobat JavaScripts – Where do they go? | KHKonsulting LLC
Hello togther,
I use this wonderfull script and want to modify it a little bit by changing the first and last Page (TitleandBacktitle).
I use the following code for this found on Adobes Webside:
app.addMenuItem({ cName: āReverseā, cParent: āDocumentā, cExec: āPPReversePages();ā, cEnable: āevent.rc = (event.target != null);ā, nPos: 0
});
function PPReversePages()
{
var t = app.thermometer;
t.duration = this.numPages;
t.begin();
for (i = this.numPages ā 1; i >= 0; iā)
{
t.value = (i-this.numPages)*-1;
this.movePage(i);
t.text = āMoving page ā + (i + 1);
}
t.end();
}// JavaScript Document
Using the script standalone is runs perfect but I want to integrate it in the splitpages script. I“m not able to get this run because I“m not so fit in Java.
So is it possible to combine this scripts?
Another Question is how to compare the original PDFname I always get a Acrobat *.tmp file so I have always to rename the Pdfs manually
Thank you very much
I use Acrobat X Pro (10), there is a available version for that ?
Nyx, the problem with Acrobat X is that there is no Document menu anymore. You can easily fix that by replacing “Document” with “File” – this will put the menu item under the File menu.
It’s works, thanks so much !!!
Pingback: Page Splitter Redux - KHKonsulting LLC
This script looks great! Thank you very much!
Do you happen to know how I could use this script on a Mac running OSX 10.9 with Acrobat X Pro?
Thanks for your help!
Kind regards,
df
David, Acrobat JavaScripts will work on both Windows and Mac versions of Acrobat.
Pingback: Splitting PDF Pages | Karl Heinz Kremer's Ramblings
the split pages script works for me, but the Reorder one produces an error. I don’t even see a menu item called Reorder Pages. In the console it says there is an error in line 20 (which seems to be: seems to be this line? nStart: i * 4 ā 3)
Error: SyntaxError: illegal character
Help?
Tom, There are some extra characters (or wrong characters) that get inserted when you copy&paste from the comment section. I’ve fixed up the script, and uploaded it. You can download the script from here: http://khkonsulting.com/files/blog/reorder.js
Hi. Got the Action for splitting working perfectly in Acrobat X1 pro.
But not able to run the reorder script. Although I have a computing background , but
I am completely new to running javascript in Acrobat.
Can the reorder script also be made into an action?
I will try and learn more about running javascripts in Acrobat.
Many Thanks
Haze77: Which script are you referring to? Anything that was described in the comments can be converted to an Action.
Thank you so much! Once I could figure out how to run it, it worked perfectly….
(For me, it was changing the “document” to “file” in the js to put the trigger on a menu… couldn’t figure out how else to run the js from within Adobe, but I’m not an Adobe expert by any stretch!)
The script was written for Acrobat 9. Adobe changed the user interface between 9 and X, and the “Document” menu is no longer available in Acrobat X and XI. That’s why you had to change the menu. I usually put things into the “Edit” menu.
Script works for me under Acrobat XI standard as far as splitting the pages is concerned. But the bookmarks in the original document are lost. Any way to retain those?
The existing bookmarks point to a page with two pages on it. In most cases, a bookmark is meant for the “left” or “right” page. The script would have no way of knowing which page is the correct page.
If the script could duplicate the bookmark for both split pages, I think it would be easier to delete the duplicate/incorrect bookmark rather than create all of the bookmarks from scratch.
Because we are creating a new document, the bookmarks are lost. Here is the problem with bookmarks: A bookmark does not necessarily point to a PDF page. It can point to a web site, it can be a trigger for something else, it can submit a form, … And, to make things even more complicated, we don’t have access to whatever action is configured for a bookmark from within JavaScript. This means that there is no simple way to recreate the bookmarks tree in the target document. If you know that all your bookmarks are pointing to PDF pages, you can try to figure out what they are by executing the bookmark action using JavaScript, and then determine what page you are on. This allows you to at least create a new bookmark that goes to that target page (or, when you create it in the new document, to one of the pages it originally pointed to).
I just stumbled across this and found the Split javascript very useful. (Thanks!)
Is there a way to “unsplit” them āĀ that is, to rejoin the two halves back into whole pages, as they were in the original document? (That is, impose them 2-up.)
I thought that was what the “reorder” script was meant to do, but it seems to be for some other purpose: when I use it on Acrobat XI, the resulting document has half pages, but now in a very mixed up order. (Not sure what sequence it’s meant to emulate.)
Thanks.
Victor, “unsplitting” these pages would be considered “imposing” them. This is best done using dedicated imposition software. I use Quite Imposing Plus (http://www.quite.com) for this purpose. This is an Acrobat plug-in that requires Acrobat to be installed. There are also stand-alone tools you can use. Unfortunately, there is nothing that’s built into Acrobat that would allow you to do that without having to “refry” your PDF documents (that’s what printing a PDF file to another PDF file is called, and it’s usually a bad idea).
This is fantastic stuff! I’m getting very close to finding a solution to a project I’m working on. I’m trying to build a personal database of my PDF textbooks (text-editable, non OCR) and manuscripts, and I’m looking for automated workflow to create smaller chunks of text from larger documents. I can easily split using ‘Top-level bookmarks’ so a 20 chapter textbook gets sectioned into manageable bits. Taking this idea further, I’m wonding if I can apply a bookmark to subheadings and thereby split the chapter into new even smaller documents consisting of only a few paragraphs. I’m not so interested in the illustrations, but capturing the text that goes along with, even if it’s filtered separately would be fine. What I am interested in is keeping separate columns and paragraphs that move from one page to another, together. Ultimately I’m going to put all of these smaller snippets into my DevonThink database, and if I do a search, and pull up a file, I would like the naming scheme for that file to maintain the hierarchy of the chapter, heading, subheading, and page number, from the original source.
Can you point me in the right direction?
Cheers,
bt
Awesomeness! So glad I found this.
Benjamin, splitting content on a page into different “chunks” is a more complex job than just splitting a document on page boundaries, and cannot be done with just JavaScript: You would need a custom Acrobat plug-in to accomplish that.
Hi,
I know this isn’t helpful, but I get on Acrobat Pro V11.0.11 “An internal error occurred.” when splitting pages. This is right after it creates a tmp file. Any troubleshooting ideas?
Thanks!
You can use the JavaScript Debugger to figure out what’s going on. Once you know where the error occurs, you can then try to find out what the variables are set to and what’s causing the internal error.
Not sure exactly what I changed to make the script work in Pro XI, but I went to preferences and clicked on all the enable… boxes except interactive console. Then it worked.
Sorry, forgot to mention I was in the JavaScript category in Preferences.
Hey Karl, this widget is amazing, just what we are looking for and works better than some paid programs!! Thank you very much!
We will need to be running this batch on a bunch of documents, so I have been trying to get it to run as a java script through an Action. I insert the code into the execute java prompt when creating an Action, but when I run the Action nothing happens. Is there something that needs to be changed to run this as an Action?
Dave again,
I see both questions answered in another post you wrote,
Thank you,
Dave, glad that you found the information.
Thanks Karl, I’m happy I found it as well, thanks for writing!
One more question, I have a funny batch of PDFs that I am splitting and need to move the page that comes out as the first page be the last page. All other pages stay the same.
I am curious if there is a way to tweek the two supplementary java scripts posted above (the ReversePages or Reorder script) to suit this function. This would be a separate item, and something that I would implement after the page split script.
Thanks for any pointers, I am pretty new to this game.
Dave, take a look at the “Doc.movePage()” method in the Acrobat JavaScript API documentation: http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_API_AcroJS%2FDoc_methods.htm%23TOC_movePagebc-76&rhtocid=_6_1_8_23_1_75
So, to move the first page after the last page, you would use this code:
this.movePage()
The good news here is that the defaults for the two parameters are already set up so that it moves the first page after the last page.
I was coming here to write that I found people writing about a java command that looks like it can help (pageMove) and I see you beat me to it!
Now I just have to learn how to code in java! š
Thanks Karl, I will l see how I can make this work.
Dave, we are not talking about “Java” here, but about “JavaScript” – similar name, but completely different programming language. If you want to learn about JavaScript in Acrobat, there is a pretty good ebook available: http://www.acumentraining.com/QEDGuides/QED_Descr_AcroJS.html
Okay Karl, thanks for clarifying.
For now I just have to work out how to use the movePage command so I can get my boss what she wants. But who knows . . .
Karl, it works!
I added this line to your Action, newDoc.movePage(0), and bingo!
Not sure how I got that, but happy it works.
Thanks for the help!
Is there a way to tinker with the value or the crop, say for example to make the crop a little more or a little less than half the page, or to crop the top or bottom of the page?
Dave, you can modify the crop box using the Doc.getPageBox() and Doc.setPageBoxes() methods:
http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_API_AcroJS%2FDoc_methods.htm%23TOC_getPageBoxbc-52&rhtocid=_6_1_8_23_1_51
http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_API_AcroJS%2FDoc_methods.htm%23TOC_setPageBoxesbc-100&rhtocid=_6_1_8_23_1_99
Just keep in mind that all dimensions are in points (72pt is one inch).
Thanks again, Karl.
I’ve been using your script for a while, I only need it occasionally, but it has worked very well in the past. Thanks
Today I keep getting “An Internal Error Occurred” when I try to run the script. I recently had an upgrade to acrobat installed – v11.0.15 – is it possible something’s changed?
Cheers,
Mario
Mario, if nothing else changed, then it’s very likely that the update is causing the problems. Are you getting any errors besides this “internal error” on the JavaScript console?
Hi Karl, I’ve been reading through your site, trying to help myself, and found the JavaScript Security “Enable Menu Items” was not checked. (It’s worked in the past, but again I don’t use it a lot and I may not have run it since installing Acrobat XI and I’ve also installed Windows 10 recently. So quite a few things may have changed.)
Anyway, I’ve rerun the script after enabling the security option and I no longer get the Internal Error. It took quite some time to run the script, but it did finish. Saving the resulting file didn’t seem to work though. I saved the file to the desktop, the window stayed up for a while, then disappeared, but the pdf file never appeared on the desktop. (Voodoo, because it did show up when I did a search. I dragged it from the search window to the desktop and it was there. I’ll have to try that again later.)
More Voodoo – I then tried running the action which I also found while poking around. That took a much longer time to complete – but it did complete. The resulting file was 22GB – the original was only 115MB, so it created a file that was about 200 times larger than the original – I’ve never used the action before, and I’m not likely to if that happens a lot. (The javascript created a file roughly the same size)
It’s late here, I’ll look into it some more tomorrow if I have time, For now I got a useable file so thanks again for the script.
Cheers,
Mario
Mario, the file size created by the Action should be similar to what the plain JavaScript created. Without seeing the file, it’s impossible to say what happened. One thing you can do is bring up the PDF Optimizer (File>Save As>Optimized PDF), and then click on the “Audit Space Usage” button to see what elements are using how much space in your PDF file.
I re-ran the action and again it produced a filed that was 200 times larger than the original. I them used the “Audit Space Usage” button on the new file as you suggested. Everything Grew in size:
Images 117,021,776 to 1,460,985,214
Content Streams 14,626 to 29, 573
Fonts 92 to 18, 754
Document Overhead 29,926 to 52, 242
X Object Forms 53,248 to 667,584
Cross Reference Table 11,860 to 792,581
Total 117,131,528 to 1,462,545,948
When I saved the optimized PDF it was back to the size it should have been.
OH – BTW – I also figured out what went wrong with saving the file with the script…I’ve got some issues with my library links it seems…so that’s a non-issue.
Thanks,
Mario
BTW, if it’s just me, please don’t worry…I’m happy using the script. I only bothered you because I thought it stopped working.
Hi all,
Thanks for writing the script. I use lots of javascripts in Indesign but haven’t used them in Acrobat. I’m using Acrobat DC now (the CC version) on a Mac. Can you please tell me where to store the splitpage.js script and how to run it, if it works with the DC version? I couldn’t find that info in this thread.
Matt, take a look here for information about newer versions of Acrobat: http://khkonsulting.com/2014/04/page-splitter-redux/ – this should also work in Acrobat DC. On Mac OS, I would recommend that you install the script in this folder: ‘/Macintosh HD/Users//Library/Application Support/Adobe/Acrobat/DC/JavaScripts/’
Wouldn’t it just be easier to duplicate every page (which I assume can be done in one step by selecting all page), then use the Crop page tool, which allows you to do “all pages” or “all odd” and “all even”. You can proceed to do the “first” page selecting the left half portion of the double-page page, then use the Crop tool for the right-hand side pages.
Robert, that is exactly what my script does, just without the additional mouse clicks. You can certainly do this manually, but if this is something you have to do on a regular basis, automating these steps will save some time. And, if you are not too familiar with the Acrobat user interface, having a script makes this task very easy to accomplish. Pretty much everything you can do in a script can be done manually, but that does not necessarily mean I want to do it that way.
Thanks for the script, it’s exactly what i needed.
Thank’s a lot for the script!
But I am facing a problem. While splitting Right to Left Arabic books, the script is rendering page number as following: 2,1,4,3,6,5……
How could one alter this script to accommodate right to left script?
I don’t know programming.
Thanks again in advance.
All you may have to do to get this working is switch the cropLeft and cropRight crop boxes around.
Thanks so much for sharing this post. I had no idea that Acrobat was extensible via JavaScript, let alone that it was so easy to work with. Looking at this now in 2020, just wanted to give you a quick heads up that while using Adobe Acrobat Pro DC I was getting an “Internal Error” when I tried to run your script (and after moving it to the Edit menu, instead of the long-gone Document menu). After seeing your tip about enabling the JavaScript Debugger, I was able to more clearly pinpoint the problem. It seems the call to `newDoc.insertPages` is now a privileged call as well. So I simply needed to move down the `app.endPriv();` line to after the initial while loop, then it worked like a charm.
Gordon, I am glad that you figured it out. Another option is to select “Enable menu items JavaScript execution privileges” in Acrobat’s JavaScript preferences.