One of the questions I get asked again and again is how to validate a field value in an AcroForm with a custom validation script. Adobe provided a lot of infrastructure to do that with just a simple script.
Let’s take a look at how to do that with a text field that is only supposed to have a value of either ‘AAAA’ or ‘BBBB’ (yes, I know that this does not make much sense in a real PDF form). So, if the user enters ‘01234’ we should see an error message that would instruct the user about what type of data is valid for this field.
To start, we create a text field and bring up the properties dialog for the field. Then we select the “Validate” tab to see the validation options:
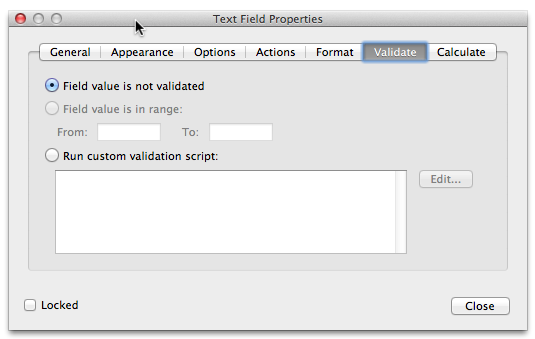
The default is that the field will not get validated. For numeric fields, there is a convenient way to validate a value range, but we want to select to run a custom validation script. After the “Edit” button is clicked, a new window will open that allows us to edit the new script:
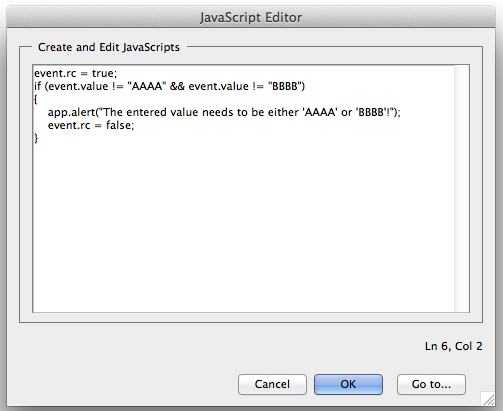
To make things easier to copy&paste, here is the script again:
event.rc = true; if (event.value != "" && event.value != "AAAA" && event.value != "BBBB") { app.alert("The entered value needs to be either 'AAAA' or 'BBBB'!"); event.rc = false; }
This script also includes a check for an empty string, so that the user can wipe out a wrong string and start from scratch.
As I mentioned before, information is passed to the validation function in the event object, and in the code we see that the member ‘value’ is used to communicate the current value of the field. The member ‘rc’ (or return code) is used to communicate back if the validation was successful or not. In the latter case, we set rc to false, and also display an error message.
When you play around with the function, you’ll notice that the validation function is only called when the focus leaves the field, so you have to click outside of the field to actually make that error message pop up. In that case, the previous value of the field is restored, and the user has to enter the data again.
This is not always desired (for more complicated data, it will probably be much easier to take a look, correct that one typo and continue with the rest of the form), so my preference is actually to mark the field so that the user knows which field needs to be corrected, and have the validation script not report a validation error back to the field:
event.rc = true; if (event.value != "" && event.value != "AAAA" && event.value != "BBBB") { app.alert("The entered value needs to be either 'AAAA' or 'BBBB'!"); event.target.textColor = color.red; } else { event.target.textColor = color.black; }
Using this method has implications on the form submission process: The form no longer can verify that the data is correct, so the submission function needs to do another round of validation to see if any of the required fields are not correct (one way to do that is to test all relevant fields to see if the text color is using the error color, or we can use global variables to store the validation state).
Another thing I like to do is to display the validation error message on the form in an otherwise hidden field: The problem with our last solution is that if the user saves a partially filled form, and picks it up at a later time, that error message that popped up is long gone, and the only indication that there is something wrong with the form is the modified field color. So, having a text field contain that error message might be a good idea.
There are other ways to highlight the field in question besides changing the text color, the border color or the fill color could be changed instead, or in addition, just make sure that you are not making the form impossible to read.
To learn more about the event object, take a look at http://livedocs.adobe.com/acrobat_sdk/10/Acrobat10_HTMLHelp/JS_API_AcroJS.88.560.html – make sure to click on the button in the upper left corner to display the navigation pane if it’s not shown automatically.
About AAAA BBBB validation
This works nicely. Just what I need. Thank you.
I was wondering what event value I would have to add to make sure that the error message doesn’t crop up when you delete an entry. Is there such a value as Null?
You can certainly test for the empty string as well:
if (event.value != "AAAA" && event.value != "BBBB" && event.value != "")
There is a null value in JavaScript, but in this case, you will actually get a valid string back from the field – it’s just empty.
Hi Karl,
Thanks for this post… Do you know where I can get some info to create a validation script for a field base on the status of a checkbox field? Or how to set a required field only when a check is enable in another one.
Thanks!
Jean-Claude,
I need a little more information: How do you want to validate the field that is based on a checkbox? A checkbox is either checked or unchecked – what do you want to do in your field? From your short description, it sounds like you want a custom calculation script to assign a (calculated) value to the required field.
Does that help?
Karl,
I have figured out how to get it. I need to see if a field (2 checkbox) was enabled, if yes, than a special discount was entered in an hidden field and use for calculations elsewhere. This look like this and works:
var cPackages = ‘Packages’;
var cReg_Price = ‘Reg_Price’;
var cDisc_Price = ‘Disc_Price’;
var nPackages = this.getField(cPackages).value;
event.value = this.getField(cDisc_Price).value;
if(nPackages == “Off” ) { event.value = this.getField(cReg_Price).value;}
By the way, just want to thank you for your blog and help on acrobatuser.com community. Your input are invaluable.
Thanks!
Jean-Claude
I am creating a form that calculates charges.. If the Invoice total that is entered manually doesn’t match the calculated total I want an error message to populate .
Can you assist with a script for this?
hi guys,
i am a newbie to coding and i am having some trouble
validating a field call emall address
i am creating a pdf fillable form and need the email field to be validated
i used this code but for some reason i cannot get the field to be validated
i am using this code please guys some help is needed here and its frustrating
function validateForm()
{
var x=document.forms[“myForm”][“email”].value;
var atpos=x.indexOf(“@”);
var dotpos=x.lastIndexOf(“.”);
if (atpos<1 || dotpos=x.length)
{
alert(“Not a valid e-mail address”);
return false;
}
}
Hi
I wonder if you would be able to help me: I have created a form using Adobe Acrobat X Pro (operating system is Windows 7). On one of the pages users have to complete a minimum of 12 out of 19 questions (these are all text fields). Is it possible to verify that the minimum number of fields have been completed? If so, please let me know how. p.s. I know hardly any Javascript.
Many thanks!
Melissa
Melissa, without knowing more about your project, it’s hard to give you advice – especially when you don’t know much about JavaScript. The solution would definitely be based on JavaScript. What I would do is create a new field that provides a status report (e.g. “You’ve completed x out of y required questions”). And then create a calculation script for that field that would evaluate how many questions have been answered. How this is done depends on what kind of input you are asking for (e.g. radio buttons, checkboxes or text fields).
Can someome please provide me with the full validation script for testing if certain fields that need to be filled out are left blank upon tryong to print. There has to be a way to since i am not submitting a form just print button. Like I have a couple of text fields, email field and a phone number field
.
Thank you!
I am looking for details on where acrobat stores the information regarding a text field, what rules should be followed and can we extract that information. So, can you help or please direct me to the right content.
You’ll find all that information in the PDF spec. If you need more information, get in touch with me via email.
Thanks.
Is there a way to validate a field to either accept “NFS” (not for sale) or a value > 0, using a custom validation script in Acrobat XI form?
I would like to validate a field to confirm if the user’s entry CONTAINS a certain word or phrase. In other words, the user may enter “My dog is brown” or “I don’t own a dog.”.
I would like to validate the field for the word “dog”
Can someone help me with a script?
Scott, I just posted a script that validates a substring on AcrobatUsers.com: http://answers.acrobatusers.com/How-I-search-text-field-substring-q119815.aspx
You are failing to set event.rc = false; in your second example when validation fails.
This is actually intentional. See the text just before the example:
So I am intentionally reporting back that no error occurred, but I am changing the color of the input field.
Great skills. Thanks for posting. I’m trying to validate a date field and want to simply drop the current date into the field if the user enters the letter “d” ( for default date). Is there a way to set validation to do this?
You can certainly do that. Try for example the following script:
event.rc = false;
if (event.value == "d") {
event.value = util.printd("mm/dd/yyyy", new Date());
event.rc = true;
}
else {
var d = util.scand("mm/dd/yyyy", event.value);
event.rc = (d != null);
}
I’m trying to restrict an PDF form Text box to exclude any numbers.
Dan, here is one way to do this:
var reg = /\d/;
event.rc = !reg.test(event.value);
This will allow anything bug numeric digits. If you want to limit your input to only letters and e.g. a space character, use this:
var reg = /^[a-zA-Z ]*$/g;
event.rc = reg.test(event.value);
This code uses JavaScript’s regular expression functionality, so if you need to adjust one of these scripts, read up on regular expressions.
Dan, great tips here, thanks very much.
In your response to Bill you provide a script that enters a date when the user types the letter ‘d’. I’m looking for a similar script that will validate the date entered by the user so that it is greater than today’s date, but less than a specific date in the future, for example 1/1/2017.
I have a field box where two values (“Estimated_Monthly_Cost” and “SB_Hidden”) are added together. If the second value, “SB_Hidden” is blank, then I need two things to occur:
– The box should not show the “Estimated_Monthly_Cost” value, and
– The box should not read $0.00. It should just be blank.
Here is my current script that doesn’t show the “Estimated_Monthly_Cost”, but does show $0.00:
var a = this.getField(“SB_Hidden”);
if (a.valueAsString != “”)
{event.value=”Estimated_Monthly_Cost”+a.value;}
else
{event.value=””;}
Any help you can give would be greatly appreciated! Thank you!!
Hello,
I need help with pdf textbox:
I want to display a warning message and highlight the textbox called “O-UIC” in my form called “IHRequestServicesForm2014” when the requestor click the Submit button. I tried the code below and it is not working:
function validateForm() {
var x = document.forms[“IHRequestServicesForm2014”][“O-UIC”].value;
if (x == null || x == “”) {
alert(“UIC must be filled out”);
return false;
}
}
I really appreciate for the help
Peters
I started with this code and substitute ‘AAAA’ and ‘BBBB’ with a Regular Expression. My problem is that when I use the following in my JavaScript code:
var myVarExp = /dog/;
entered within the Variable tab of the Text Field Properties dialogue box – it does not see it as a Regular Expression.
That is to say; if I type ‘dog’ (ignore quote marks) into the live pdf field it gives an error, but if I input ‘/dog/’ (ignore quote marks) into the field it works fine.
My Question: why is my var not being recognized as a Regular Expression.
I had read that the open ‘/’ and close ‘/’ (ignore quote marks) defined a Regular Expression but this does not appear to be case. What am I missing here?
ffot – just declaring a variable as a regular expression does not test the value for the expression, you need one more line:
var re = /dog/;
event.rc = re.test(event.value);
I have the same issue as “Ellie” above. I have a subtotal field that reads $0.00, when it should be blank when there is no value above zero.
Thanks.
This is done via a custom formatting script. The simplest way to do that is to use these two lines:
if (event.value == 0)
event.value = "";
Thanks Karl,
It still didn’t zero out the field. This gets inserted under the validation section under “custom script”, i don’t need to define any variables, etc?
Shelby: No, this is not a validation script, the last script I provided is a custom FORMATTING script, so you have to go to the formatting tab and select to use a custom script.
Oh man newbie alert. Thank you!
I am trying to make an invitation for my tita. I am not a programmer at all. However, the invitation I made has text boxes that lets the user enter how many adults and kids will attend. Then they have a choice of beef, chicken or fish. How do I validate these fields (by itself or combined) so that the number they enter will not exceed the number of attendants entered?
Thank you so much. Please just send me the codes! hahahahaha!
I am trying to require that a specific number of number characters (not just limiting the field to a certain number) be entered into a pdf form field. I thought the script provided above would work but I am having to enter the exact numbers that I place in script if I change the AAAA and BBBB with 1234. If I change the numbers 1234 out in the script for #### I have to enter #### rather than actual numbers to get it to not error. This was how I was changing the code. Please let me know where I am going wrong.
event.rc = true;
if (event.value != “####” && event.value != “####”)
{
app.alert(“The entered value needs to be either ‘####’ or ‘####’!”);
event.target.textColor = color.red;
}
else
{
event.target.textColor = color.black;
}
I am thinking that I don’t need the BBBB section of this code because I simply just want the user to be required to enter 4 numbers into the field.
Thanks for any help you can give me on this.
You can use for example this code to test if the value is between 4 and 5 characters long:
event.rc = true;
if (event.value.length >= 4 && event.value.length <= 5) { event.target.textColor = color.black; } else { app.alert("The entered value needs to be either 4 or 5 characters long!"); event.target.textColor = color.red; }
Thank you for getting back to me. What if I want the box to be exactly 4 characters?
Karen, change the 2nd line in my code to this:
if (event.value.length == 4)
Thank you so much for your help. You have saved me a tremendous amount of time!
Would you be a source to help me with another submit button javascript problem I am having or is there another forum I should use?
Thank you again-
Karen, if you need professional help, please contact me via email (my email address is on my “About” page), if you are looking for free help, please post your question in the AcrobatUsers.com “Answers” section.
hi karl,
need help with a small script on acrobat where people cannot save the pdf file without filling up the required fields?
thanks in advance
@Phil: You cannot do that. The save function always works, and in my opinion, it’s a good thing that it aways works: I may start to fill out a form, but may need more information. In this case, I would save the document (and potentially leave it open, but I want to make sure that the information I’ve already entered cannot get lost), retrieve the information and then continue. I may even close the document if I know that it will take a few days to get the missing information. So, preventing somebody from saving your form is a bad idea, and from a technical point of view also not possible. What you can do is prevent somebody from submitting your form if it’s not complete. This is done by either marking the text fields that need information as “required”, or by providing your own validation mechanism that gets executed when the user clicks on the “Submit” button. What exactly you do to validate the form depends on your specific form, and there is no general purpose function that you can use.
Your right Karl save button must always be there
thanks again
I was looking around to see if we can trim or crop out part of a field after the first leading space.
ex.
user input = 114 Main street, the results would be 114 the rest would be trimmed off.
I would also want to do it in reverse, keep whatever is after the first space.
is that kind of validation possible on PDF?
Steve, that can be done, but it would be via a formatting script, and not through validation.
How can i restrict user from printing if the required field is blank?
I created a button and input the below code, but it doesn’s stop printing after the alert message prompt out. besides, I want to create an alert message when user click on the original ‘print’ button of PDF ( not the one I created). May I know how ?
ray=[];
for(var i = this.numFields – 1; i > -1; i–)
{
var fieldName = this.getNthFieldName(i);
if (this.getField(fieldName).type==”text”||this.getField(fieldName).type==”combobox”||this.getField(fieldName).type==”radiobutton”)
{
if ((this.getField(fieldName).required==true)&&(this.getField(fieldName).value==””))
{ray.push(this.getField(fieldName).name)}
}
}
if (ray.length>0)
{app.alert(“Some required fields were not filled in. Please complete all required fields.”)}
else
{this.print(false)}
Jackie, you cannot prevent printing. When the user uses the “Print” button (or menu item), the document will print. What you can do is hook into the “Will Print” document action and run your validation step and then display the message, but the document will print.
What I do in a situation like this is to mark up the document so that it’s clear that it is not a valid document. you can do that by e.g. creating a hidden field that covers the whole page and gets only shown when the validation fails. You can then hide the field again during the “Did Print” document action.
I used this formatting script you recommended in a previous post so a field would appear blank if the value is 0. Worked great. However, if the value isn’t 0, how do I format it to include a “$” before the number?
if (event.value == 0)
event.value = “”;
Mary, that needs to be done with a custom “formatting” script, not a validation script (which is what this post is about). To prefix a “$”, you can use this:
if (event.value == 0)
event.value = "";
else
event.value = "$" + event.value;
Hi Karl
I have the following problem which I can’t figure out with my limited JS.
I have two text boxes, this is the code
var n = this.getField(“Actual”).value;
var m = this.getField(“Allowed”).value;
// check for exceedance
If ( n > m ) app.alert (“you have exceeded”);
I get a syntax error, please help? Thanks so much
Graeme, what syntax error are you getting? From how your code is pasted here, I cannot tell if you are using the correct quotes. Make sure you are using the standard quote, and not any “fancy” quotes from e.g. MS Word. Don’t edit your script in a word processor, edit it either in the Acrobat JavaScript editor, or in a text editor.
Hi Karl
Thanks I sorted that out;
Can you help with the following;
I have 3 text boxes to do a duty calculation. This code is in the calculate section of the Sign Off text box. I want the text in the adjacent text box to change to red if n > m below
here is my code;
//Set the vars n and m
var n = this.getField(‘Actual’).value;
var m = this.getField(‘Allowed’).value;
if( n > m ) {
app.alert(“WARNING! You have exceeded your Duty”);
}
// I want the ‘Actual’ text field to change to Red Font if n > m above
Can you help?
Thanks kindly
Use this code:
//Set the vars n and m
var fActual = this.getField("Actual");
var n = fActual.value;
var m = this.getField("Allowed").value;
if( n > m ) {
app.alert("WARNING! You have exceeded your Duty");
fActual.textColor = color.red;
}
else {
fActual.textColor = color.black;
}
This will either set the text color to red in the case of m>n, or back to black.
Hi Karl
I would like to have a script on a textfield called account restrictid to only numbers, dash – and space, like that the user can typ in 1111 22 33333 or 1234567-8, im using AcrobatX and i cant see any way to custom this without a script.
Another way could be that if there are 2 – 8 number, a popup will ask if user will insert a dash before the last number, then user could answer yes or no, or if number are more than 8 number, no question would popup.
I guess the last choice would make a much more complicated script?
Good morning Karl,
Is there any way i can have a text box mark as read only after a pdf form is saved.
i know i can used the digital signature , but i would like to know if there is another way.
Thanks in advance
Kent, you are right, the last case would make for a more complex script. To create a field that allows digits, dashes and spaces, you can use the following custom keystroke script:
if(!event.willCommit) {
// Test individual keystrokes and clipboard pastes
event.rc = !isNaN(event.change) || event.change == ".";
} else {
// Test the entire pre-commit value
event.rc = !isNaN(event.value);
}
I assume you would want to change the text box just before it is being saved, so that the modified version is saved to disk. You can do this using the document event “WillSave”, but I think this would get in the way of the user: I am so used to saving documents that I am working on while I am editing them. Your event handler for “WillSave” would then change the text box to read-only, just because I did not trust the application to not crash while I was filling out a form. So, just saving a document should not trigger such a change. You could do this when the user selects to “Submit” the document.
Thanks for the quick reply , what java script i need to used in the event for ” WillSave”
thanks
Eddy, the most straight forward script would be this:
this.getField("FieldToChange").readonly = true;
Karl do you have an email so i can ask you some more question.
Eddy, my email address is on my “About” page (http://khkonsulting.com/about/).
Hi Karl
Thanks a lot, the only change i did was to replace event.change == “.”; with event.change == “-“; to have it work.
Thanks a lot for your help.
Karl thanks a lot, everything works
Kent, sorry about that. I was copying and pasting and picked up the wrong code snippet.
Good morning Karl,
I would like to create a check box which when clicked would strikethrough (RedLine)the value of a text field. I have the following script.
textfield.font.lineThrough = “1” but it does not work . on adobe acrobat pro. can you please help me
thanks
Eddy, that’s not possible. I don’t know where you found that code snippet, but that’s not something that would work with a text field. When you look at the Acrobat JavaScript API documentation, you’ll find that a text field (of type “Field”), does not have a “font” property, but a “textFont” property, which is not an object, but a simple string that contains the font name.
Hi Karl thanks for your help
I have another question related to time. If I have a text box where you input a start time in HH:MM and then another textbook for a end time in HH:MM, how do i get it to calculate properly if it goes past midnight?
Hi Karl I have some questions. First I am trying to change the contents of a text box depending on 2 other fields. the box to change is called Shipping and the to other fields to check are subtotal and customer #. For the shipping to be any # the other 2 fields have to meet a criteria. First the sub total has to be less that $250.00 then the customer # will start with 1 of 3 things CA, UT, or CO. IF both conditions are met then the shipping field will show “$9.00”. If the subtotal is less than $250.00 but the customer # does not start with one of the three items then the shipping field should show “$11.00”.
If the subtotal is $250.00 or above, then no matter what the customer number starts with the shipping will show “$0.00”..
Note I have a check box that upon checked will change the shipping to “waived” if i chose to waive the shipping. I have some code but as you will see i am stuck on a few things.
if this.getField("Sub Total").value < "250.00" && this.getField("Customer #").value starts with "CA" || "UT" || "CO" {
this.getField("Shipping").value = "$9.00"
}
else if this.getField("Sub Total").value = "250.00" {
this.getField("Shipping").value = "$0.00"
}
Sorry some of the code was cut out. Here it is again
if this.getField("Sub Total").value < "250.00" && this.getField("Customer #").value starts with "CA" || "UT" || "CO" {
this.getField("Shipping").value = "$9.00"
}
else if this.getField("Sub Total").value = "250.00" {
this.getField("Shipping").value = "$0.00"
}
It looks like the same code that you’ve posted before, and because the first line is not valid Javascript, I assume that the problem happened again. Please get in touch with me via email (my email address is on the “About” page – http://khkonsulting.com/about/ )
Hi Karl, I am using the code below but it doesn’t seem to work correctly. I have a text box start/date time and another end/date time. The result should be in a decimal format eg: 5.25
I have to include a date as the start time might be 20:00 today end end after midnight 02:00 tomorrow. Is this the easiest way to do this?
function Date2Num(cFormat, cString) {
// convert cString with cFormat to number of minutes from Epoch date
// convert to date time object
var oDate = util.scand(cFormat, cString);
// convert date time object to minutes
return Math.floor(oDate.getTime() / (1000 * 60));
} // end Date2Num function
// format for inputted date & time
var cDateFormat = “m/d/yyyy HH:MM”;
// field names
var cStartField = “Start Date/Time”;
var cEndField = “End Date/Time”;
// get field values
var cStart = this.getField(cStartField).value;
var cEnd = this.getField(cEndField).value;
// clear the result value
event.value = “”;
// compute the difference in minutes if there is data
if(cStart != “” && cEnd != “”) {
var nDiff = Date2Num(cDateFormat, cEnd) – Date2Num(cDateFormat, cStart);
// convet to hours
event.value = nDiff / 60;
}
You will have to test to see if the end time is smaller than the start time. However, in your case, this should not be a problem, because you also take the date into account. So, if I enter “1/1/2015 20:00” and “1/2/2015 2:00”, you should get the correct output (6). What problems are you running into?
Hi Karl
I keep getting a Syntax error: missing ; before statement ? When using the above code ?
I just have 3 text fields, Start Date/Time, End Date/Time and Total. I am a little unsure of what code goes where?
Hi Karl
Problem Fixed. I found your solution to the same query on another form. Thank you
// Time Values
var cStartTime = this.getField(“OffChocks2”).value;
var cEndTime = this.getField(“OnChocks2”).value;
// Only process if field contains a value
if ((cStartTime != “”) && (cEndTime != “”)) {
// Convert to Hours Decimal value
var nStartTime = 0,
nEndTime = 0;
var aStartTime = cStartTime.split(“:”);
nStartTime = Number(aStartTime[0]) + Number(aStartTime[1]) / 60;
var aEndTime = cEndTime.split(“:”);
nEndTime = Number(aEndTime[0]) + Number(aEndTime[1]) / 60;
// Find Difference
var nTimeDiff = nEndTime – nStartTime;
// Test for Midnight Crossover
if (nTimeDiff < 0) { // Shift 24 hours
nTimeDiff += 24;
}
// If used in a calculation, may need to be changed to "event.value ="
var nHours = Math.floor(nTimeDiff);
var nMinutes = Math.floor((nTimeDiff – nHours) * 60 + 0.5);
event.value = util.printf("%02d:%02d", nHours, nMinutes);
} else
event.value = "";
This sorted out my going over midnight problem.
How can I change the result to be in a decimal format e.g. 10.75 ?
Also how can I take a time in HH:MM and add 2 hours to the time and show that in the adjacent text field?
thanks for your help
Nice code
Can anyone shed some light?
I have a time entered in “textLocal” and I want to display UTC time converted from the local input in “textUTC”
How do I go about that?
Graeme, in general, you would use the JavaScript Date object. This is nothing Acrobat specific, so any JavaScript resource (tutorial, book, …) that shows you how to use the Date object will help you with this.
Hi Karl thanks I will look into that. How do I change the event.value result in your above code to give a decimal output like 10.25 hrs?
Thanks kindly
Unfortunately I don’t know what code you are referring to. You can get a number with two decimals when you use the util.printf() method:
// ....
// let's assume the variable num is set to 10.25
var num = 10.25;
event.value = util.printf("%.2f", num);
You can find more information about util.printf (which is Acrobat specific, so in this case a general JavaScript resource would not help) here: http://help.adobe.com/livedocs/acrobat_sdk/11/Acrobat11_HTMLHelp/wwhelp/wwhimpl/common/html/wwhelp.htm?context=Acrobat11_HTMLHelp&file=JS_API_AcroJS.89.1257.html
Hi Karl, thanks
I am using this bit of code below I found on another forum where you helped someone with the same midnight problem.
// Time Values
var cStartTime = this.getField(“OffChocks2?).value;
var cEndTime = this.getField(“OnChocks2?).value;
// Only process if field contains a value
if ((cStartTime != “”) && (cEndTime != “”)) {
// Convert to Hours Decimal value
var nStartTime = 0,
nEndTime = 0;
var aStartTime = cStartTime.split(“:”);
nStartTime = Number(aStartTime[0]) + Number(aStartTime[1]) / 60;
var aEndTime = cEndTime.split(“:”);
nEndTime = Number(aEndTime[0]) + Number(aEndTime[1]) / 60;
// Find Difference
var nTimeDiff = nEndTime – nStartTime;
// Test for Midnight Crossover
if (nTimeDiff < 0) { // Shift 24 hours
nTimeDiff += 24;
}
// If used in a calculation, may need to be changed to "event.value ="
var nHours = Math.floor(nTimeDiff);
var nMinutes = Math.floor((nTimeDiff – nHours) * 60 + 0.5);
event.value = util.printf("%02d:%02d", nHours, nMinutes);
} else
event.value = "";
It works perfectly but I just want to change the result to decimal and not HH:MM ?
I'm stuck!
The following is without any testing, so things may blow up 🙂
Change the last few lines (starting with the // If use in calculation to this:
event.value = util.printf("%.2f", nTimeDiff);
}
else
event.value = "";
It works! Thanks a million
Hi Karl,
I have a set of 5 checkboxes (each representing a specific type of report – for example Type A, Type B, etc.) Next to each checkbox is a dropdown box to select the report frequency (weekly, monthly, quarterly, etc.) I want to require that the user selects a frequency if the corresponding report type check box is selected.
Right now, I have the following custom calculation script on the dropdown box field:
event.target.required = (this.getField(“Care Management Alert”).value == “Yes”);
However, this does not prevent the form from being submitted; it just puts a small red box around the dropdown field if it’s not filled out.
Is there any way to actually put a hard stop here? Or at the very least, is there a way to highlight the entire field or somehow draw more attention to it?
Thank you much!
Lisa, the required property only works for text fields, and all it does is, it checks if the field is not empty. To come up with your own form level validation, you will have to write some JavaScript. This post was about field level validation (e.g. to require that data is entered in a certain format). Doing a form level validation is something different. Do you have a “Submit” button? If so, you can convert that to a button that calls such a form level validation script, and only executes the submit function if every requirement is met.
To highlight the field with more than the red outline, you can use the Field.fillColor property (e.g.
theField.fillColor = color.red;
), but this will only work if the user has not selected to “Highlight existing fields”. If that feature is selected, you will only see the fill color when the field is active (meaning, being edited). The best way to do this is to not allow the user to submit the form until all information is filled in. You can then display an alert that points the user to what information is missing.Thank you very much!
I tried this code and it worked but I want to adapt it to use numbers rather than AAAA and BBBB. For example, if Field A is less than or equal to field B the event is true and Field A should be displayed as entered. If false, I want the app.alert to appear. How would I do that?
event.rc = true;
if (event.value != “AAAA” && event.value != “BBBB”)
{
app.alert(“The entered value needs to be either ‘AAAA’ or ‘BBBB’!”);
event.rc = false;
}
Hello Karl
I am looking for a basic validation code for entering information such as a telephone number on a form so that only 10 numbers are entered, but the result is formatted as XXX-XXX-XXXX. I notice that the Field Properties box has a Format tab and a Validate tab. Would a different code have to be put in each area? Thanks so much.
David
Actually I would prefer the phone number to be formatted as (XXX) XXX-XXXX
Thanks
David
David, when you select the “Format” tab, then select the “Special” format, you can actually pick the phone number format from the list. This will take care of both setting the custom keystroke and formatting script for you. You don’t have to do anything on the “Validate” tab. The keystroke script will make sure that you only enter valid characters, and only the correct number of these characters, and the formatting script takes care of formatting the data as (xxx) xxx-xxxx. If you want to do this by yourself, you will have to provide both a custom keystroke and a custom formatting script, but you will not have to enter a validation script.
Elaine, you can do this with the following code:
// get the value of "FieldB"
var fieldB = this.getField("FieldB").value;
if (this.getField("FieldB").valueAsString == "") {
app.alert("Please fill out 'FieldB'");
}
else if (event.value > fieldB) {
app.alert("FieldA must be less or equal to FieldB");
}
However, keep in mind that this is not getting executed when somebody modifies FieldB. The better approach would be to put this “validation” into a calculation script of a hidden and read-only field. You would then be able to catch any case where – either through manual input, or via a calculation – FieldA ends up being larger than FieldB.
Good morning,
I am not a programmer and tried a basic script and it didn’t work. so what I need is i have 4 fileds that need to be mandatory and i did the required button but its not saving it properly so i figured i needed to validate them, they are first name, last name, address and email address.
Please help.
thanks
Hi Karl.
I’ve reviewed all your really helpful posts, and have tried adapting a few of your suggestions for my requirement – but can’t get anything to work..
I currently have a numbers text field that calculates a total based on two selected fields, this of course works fine using the standard preferences.
Ideally I would like the colour fill of that calculated text box to change – depending on the total it provides, i.e.
if 6 10 17 – red
Any guidance would be gratefully received.
Thanks
Jon, take a look at this tutorial on AcrobatUsers.com for more information about how to work with field colors in validation scripts: https://acrobatusers.com/tutorials/using-colors-acrobat-javascript
Hello Karl, great information thank you.
Apology if someone already asked but is it possible to do both from above? Throw an error message, turn the text red, and not allow them to jump to the next field using the tab key(keeping the text they already typed and not reverting back to previous)?
I tried playing with your example but couldn’t get what I needed to work. I have a comb field of 10 that contains a random mixture of numbers and letters. if all 10 boxes are not complete then the user gets a message telling them all 10 boxes need completing.
Anna, you can use the following custom validation script to require 10 characters:
if (event.value.length != 10) {
app.alert("The data entered needs to be exactly 10 characters long!");
event.rc = false; // remove this line if you don't want to revert the edits
}
I am creating an Adobe form & have a group of radio buttons , the last of which is “Other:” if this radio button selected I would like the accompanying “Other” Text box to become a required field.
I found a sample of a for doing exactly this, but I can’t seem to see the actions/script that makes it work. & not being well versed in programing java…
Here is the example: https://forums.adobe.com/servlet/JiveServlet/download/2551941-36280/Required%20Fields.pdf
Diana, the form you’ve linked to is a “LiveCycle Designer” form, not a “normal” AcroForm. Are you using Acrobat to create your form, or LiveCycle Designer?
I am using Acrobat, is this doable? thanks!
Greetings Karl,
I’ve found your posts to be quite helpful but I’m working on a project that I can’t seem to get working. The goal is to have users enter an email address in text box, and enter it in a second box for verification. For the first box I found the line
event.rc = eMailValidate(event.value);
and while it does seem to only allow a properly formed email address to be entered, it does not tell the user if there is a problem or leave the faulty address in tact to be corrected as your AAAA code does. I like in your example that the text is turned red and remains, so the user can fix it. How can I substitute your specific value (event.value != “AAAA” && event.value != “BBBB”) with my email string and use the rest of your validation code?
Other questions is, is there a way to validate one field to another? I’d like to have the second box give error if it is not identical to the email address entered in the first box.
Thanks again for your great directions!
Oh god this is driving me mad. This is the closest forum I’ve found, plus you reply so very quickly and I need this done as soon as possible.
If you are able to quickly piece together a Javascript code for me that would be SO helpful!
I need one button which when pressed will do a few things: first it needs to validate the form. If any required fields are not filled in it would pop up with a customised message and then highlight all unfilled fields in red.
It would then change the form into a ‘read only’ form whereby it can no longer be edited. (not flatten because I hear Adobe Reader doesn’t give access to that) Then the Save As menu would pop up for the user to save the file as a read-only PDF. Then when saved it would email it out to two addresses as an attachment.
I have everything so far but I do not know how to flag up unfilled required fields in red. Your forum is the only forum that has come close to answering it. If you could help me out I would be very much appreciative.
James, unfortunately, I cannot do custom software development for free. I can help you to do this by yourself, but if you want me to create this for you, I would have to charge you. I can certainly help you with the one task you mention you still need to do, marking up the unfilled fields. I would use the following snippet to mark a field with a red outline:
this.getField(“Text1”).borderColor = color.red;
To switch the border color back to e.g. black, you would replace color.red with color.black. For other colors, lookup the color property in the Acrobat JavaScript API documentation.
Hi Karl, I believe I got the second part of my problem figured out by using a getField and comparing the value. Not sure if this is the simplest way to get it done but it I added this in the EmailVerify field and it works great.
var n = getField(“Email”).value
event.rc = true;
if (event.value != n)
{
app.alert(“Email adresses do not match.”);
event.target.textColor = color.red;
}
else
{
event.target.textColor = color.black;
}
Still haven’t been able to get the validation to work using the eMailValidate for the variable and your code for the pop up and color effects, so any tips would be appreciated.
Brian, I don’t know what eMailValidate() is – it’s not a standard Acrobat JavaScript function. Did you add a custom script to your document? If not, you need to add a script that defines this function.
Hey Karl, yes, I had added a custom script under the Validate tab for the field…
event.rc = eMailValidate(event.value);
I read somewhere that the above string and
event.rc = CBIsValidEmail(event.value);
are “unpublished” (not sure where they got it) Acrobat code to validate the format of an email address instead of having a really long validation string with all manor of character combinations and such. Wherever I saw that post, someone else had added that the first option seems to work better so that’s the one I’ve been playing with.
It sounds like it is something more experimental than anything at this point. Do you have a suggestion how I might validate an email address with regards to it being properly formatted at least (i.e. username1@myemail.com)?
I am not aware of these two functions, and as you’ve experienced, they don’t work. What you are trying to do is just standard JavaScript, and has nothing to do with Acrobat’s JavaScript, so you can just google for “javascript email address validation”, and you should get a ton of different scripts.
Oh Karl you are amazing! Thank you very much. The last problem I have is if there are unfilled boxed the warning message now comes up and highlights it red but annoying it allows the next action to go through which is my save action. I don’t want it to save unless all required fields are filled. Is there anyway of stopping the user going to the next action if unfilled fields are up? I hope this is that last time I take up your time. Thank you so much for your help so far! 🙂
Hi Karl, wondering if you may have had time to consider my problem yet, thanks!
Diana, sorry about the delay, I missed your first reply.
Make sure that your “Other” field is named based on the name of the radio button group: Let’s say your group is “Group1”, then name the corresponding field “Group1_Other”. Then, create a document level script named “HandleOther()”:
Then, add the following as the “Mouse Up” event handler to every radio button in the group:
That should do it. It will also hide the “Other” field, so that nobody can accidentally fill it in.
Perfection! Thanks ever so much
May I ask you to assist in adding a second radio button/text box combo to the same group?
Diana, what exactly do you mean by adding a second radio button/text box combo to the group? What would the names of these “Other Too” buttons be?
My Radio Button Group is called “Referral” to collect data on how a client finds us.
Advertisement
Google/Web Search
Phonebook
Walk/Drive by
Website (with text field to specify)
Other (with text field [Referral_Other] to specify) – this one you have already helped me with 🙂
I also have one other question for you if I may – Say that after going to all the trouble of perfecting a form you then find that the document itself requires significant changes. I know that I can make minor text edits in Adobe, but things like line wrapping cause issues…so I would want to edit in word & then convert to pdf again. Can I copy oall of my form work to the new form? or am I SOL?
Thanks so much in advance!
Diana,
you can use a variation on the original code to add another “other type” field:
In the case you describe, open your original document (the one with the form fields), then select Tools>Pages>Replace and select to replace all pages with the pages from you updated document. This will just replace the static page content ‘behind’ the form fields, but will leave the form fields intact. You may have to adjust their size and position to make them fit with your updated form, but you don’t have to recreate any of the JavaScript, appearance settings, or even document level scripts.
Hi Karl,
Hope you can help. I’m creating a form with a drop-down box that allows multiple selections. However, i want to restrict the number of selections allowed to only 3 out of 10 choices. Is it possible?
Thanks
Hello Karl
i need a bit of help please. I have a pdf form in Acrobat XI. In this form i have 4 fields for subtotals ( in %) . The last field is”Total” which summarize all this 4 Subtotals fields. The problem is that i need to set this to not exceed 100% and to display an alert in case of the sum is less or more than 100%.
Can you help me please?
thank you in advance!
cesar
Cesar, you would do this with a custom calculation script in the Totals field. You are already adding up the subtotals, so if you end up with a value different from 100%, you can then display a message. Look into app.alert() in the Acrobat JavaScript API Reference. Another option would be to automatically could how many of the fields are filled out, and if it’s one less then available, automatically fill out the last one with the difference to 100%.
I have a form where I need to restrict what the user can enter. For example, my form has a text field that requires the name (or abbreviation) of each state the customers does business in. I need the name of each state, and can not accept “all over the country”, “all 50 states”, “the entire U.S.”, etc. Is this possible?
Linda, that’s certainly possible, but I would take a different approach: You can create a list box that allows a multi-selection. This way, the user can select all states that they do business in, and there is no question about what can or cannot go into that field. If you want to use a text field, you will have to do some heavy text processing to filter out anything that is not a state or a state abbreviation.
Hi Karl! might there be a script to change all form field names & Tool Tips to ‘Title Case’ aka Capialize Each Word?
Karl – that’s a perfect solution! I never would have thought of that. Thanks so much!
Dear Karl,
I faced some similar Problem: I have a Radio button called Invest, which has two Options “Yes” and “No”. This button is placed at the beginning of my PDF. At the end of the document I have some summary, where some text fields are included. One of this fields is called Invest_State and I try since several days to force Adobe Acrobat Pro to get in the field Invest_State the verbal description of the Radio button (in other words – this field should consist Text “Yes” or “No” respectively to Option choosen by the Radio button. Since I’m very new in the Java script under PDF I would like to ask You as an expert: how can I solve this Problem? I suppose -one has to calculate theInvest_State field but how to calculate something, which is not number.
I would like to ask you for some advice or some good example in web…
Thanks in advance
“Calculation” does not necessarily imply a number. You can “calculate” a full name by combining both first and last name. Open up the property dialog for both of your radio buttons and make sure that they use the export values “Yes” and “No” (this is done on the Options tab). How you can open the property dialog for your Invest_State field, and select the “Calculate” tab and create a “Custom calculation script”:
Thank you for your comment. I will let you know when I sold my Problem, best regards
Hi Karl,
I’m trying to set up a grid matrix of fields and values. The value limits are between 0 and 8 and I can use your script above to validate the values, or I can set the format to “number” and use the between values in the validate box. However I’d also like to change the background colour of the field to a custom colour and depending on the value I have inputted. For example if i type in “1” when I exit the field the background changes to green, but if I choose to update later and type in “2” the background will update to blue.
I’ve been trying to use “if” and “else if” but I can’t seem to get it to work and along with that have it tied to the value validation script. Can you help?
Matt, take a look at that tutorial (not by me, but by somebody who is one of the expert scripters for Acrobat): https://acrobatusers.com/tutorials/using-colors-acrobat-javascript
Dear Karl,
1.How to submit button works and collect data Fields in local server?
2. How to use Workflow Concept?
3.Digital Signature properties: The script executes when field is signed?
Regards
Elango Varadharajan.Kesavan
Elango, your question have nothing to do with this blog post. You may want to take a look at the tutorials on AcrobatUsers.com to see if they can help you with your problems.
Hello Karl,
I am creating a form and need your assistance.
I am trying to validate text fields 3 different ways, First i need a script to validate only letters and spaces second i need to validate a field with only numbers. And last i need to validate a alphanumeric field. have a hard time figuring this out being new to coding and all.
Thanks in Advance for your help.
Hi Karl,
1. How to data fields insert and collect from local server?
You insert form fields using Adobe Acrobat’s Form editor. To collect data on a local server, you would need to run a web server (Apache, IIS, …) and create a page that can collect a forms submission. How this is done depends on what submission mechanism you want to use.
Sherman, Take a look at this comment I posted a while ago, it should get you started: http://khkonsulting.com/2012/11/validating-field-contents/#comment-107035
Hi Karl,
I’m hoping you can help me. I’ve got a form that I want the first field (DateRow1p5) to be a date (mm/dd/yy), which I’ve set up in the formatting section. I then have 23 additional fields that I want to add one day to each of them (so field “DateRow2p5” reads 08/02/15 if “DateRow1p5” reads 08/01/15, field “DateRow3p5” reads 08/03/15, etc. The code I have used for that is:
var f = this.getField(“DateRow1p5”);
var d = new Date(f.value);
d.setDate(d.getDate()+1);
event.value = util.printd(“mm/dd/yy”, d);
The problem I encounter is when I delete the first field, I get an error for each additional field saying “Invalid date/time: please ensure that the date/time exists. Field [ DateRow2p5 ] should match format mm/dd/yy”.
I don’t know how to add javascript that will clear the fields if I change that first field (the “DateRow1p5”) without throwing up the error. If there is a better custom calculation script, I’d be interested. Any help is appreciated.
Bret, you can avoid that by adding a check that verifies that you have valid data in your source text field, and if it’s either an empty string, or if the string cannot be converted successfully to a date, you would not run the code that sets your additional fields, instead, it would set these fields to contain an empty string as well.
In a form out of 10 text boxes i have to validate 5 text boxes . How can I do that using javascript?
My question is very simple but this is the first time I create a pdf form that you can fill out. I need any field written on a certain box to always be multiplied by 4. Can you help me?
thanks
oops… I meant to say any number written on a certain box to always be calculated by 4.
Waleska, you may want to review this tutorial, it covers basic calculations in PDF forms: https://acrobatusers.com/tutorials/how-do-i-use-basic-calculations-in-a-form
How to set “validation script” with playing sound?
I need instead “The entered value needs to be either ‘AAAA’ or ‘BBBB’!” play external sound.
Please help
svito, take a look at the Acrobat JavaScript API document (you cannot program for Acrobat without having access to this document), and look for the Doc.exportDataObject() call. The nLaunch parameter will allow you to open the external file.
Sorry, this may be a silly question and I am quite ignorant about most of this but your site seems to be the only hope of getting anywhere. I am trying to structure my PDF forms so that a form field will become required if a seperate check box or radio button is checked, or drop down menu item chosen.
For example:
Q: Marriage Status A: (drop down menu with 3 choices: Married, Single, Divorced).
Q: Spouse Name
I need the form to require a spouse name in the second field, only if the answer to the first question is Married. Is there a way to do so with Acrobat Pro X?
Chris, this is often done for a dropdown that has an “Other” selection, which then enables (or requires) the “Other” text field (which is what my example is about, so you will need to adjust the selected option). You can do this in two steps: Step one is to select “Commit selected values immediately” for the dropdown control on the “Options” tab on it’s properties dialog, Step two is to create a custom calculation script for the field you want to require and use the following code:
Hello, I am brand new to coding and found this script online to validate a date range. My purpose is to allow a user to choose pay frequency (weekly, bi-weekly, semi-monthly, quarterly, annually) and subtract it from a certain date.
Let’s say I got paid on 10/16/2015 and chose the weekly option, the value for the week would be – 7 (days) to make my last paycheck on 10/09/2015 and if the same drop down menu with the bi-weekly option was chosen the value would be -14 (days) so my last paycheck would’ve been 10/02/2015. So on and so forth with semi monthly (-15), monthly (-30), quarterly (-90), and annually (0 divided by 12). I have the following formula but something is off.
I have 3 fields:
field “BB” which is the drop down menu with pay frequency and each one has been assigned a value (-7 for weekly, -14 bi-weekly and so on).
I have field “BB1” in which the user enters the last date they were paid on and so when the drop down option (pay frequency) or field BB is chosen, it will calculate it based on the date minus the frequency chosen.
I have a field titled: “P1” where it shows the date based on the formula I am attempting: “BB1” – “BB” (value chosen) to equal the date for field “P1”. I hope this makes sense. Here is the script:
(function () {
// Get date from field
var v = getField(“BB1”).value;
if (v) {
// Convert string to date
var d = util.scand(‘mm/dd/yyyy’, v);
// Subtract 30 days
d.setDate(d.getDate() = BB);
// Set value of this field to the new date
event.value = util.printd(“mm/dd/yyyy”, d);
} else {
// Blank field if no date entered
event.value = “”;
}
})();
Thank you and I truly hope this can be solved.
P.S.
I am able to do a simple method and create more boxes, I just want to simplify the drop down value and have the option for the same (if possible). Thank you so much in advance for your time and assistance! 🙂
I forgot to add there’s another simple formula that seems to work in excel but not JavaScript for adobe forms. My problem is simple:
If box “A” minus box “B” = a negative number then zero but if greater than 0 then any number to not exceed $500.
Here is my code and it works on excel perfectly but not this.
=IF(A-B<=0,0,MIN(500,SUM(A,B)))
Thank you
Hello,
I sent an email yesterday regarding figuring out a formula. Would you be so kind as to tell me what the cost would be for the solution? or “consulting fee”? Thank you
Gio, your request has nothing to do with the blog post you are replying to. What you want to do can certainly be done, but it’s a bit too much to cover in a reply here in forum. You can either contact me via email for a quote on a consulting project, or if you are looking for free help, post your question in the AcrobatUsers.com forum (https://answers.acrobatusers.com/Default.aspx).
Gio, Acrobat does not use the Excel syntax for formulas. You will have to convert your Excel formula to JavaScript. There is no easy way to do that, and whatever you will end up with almost always be longer than your Excel formula.
Gio, I am sorry for the delay, but you did not send an email – you replied to a post on my blog. I don’t usually reply right away, my customers always come first, and maintaining this blog is something I do when my workload allows it. This means that sometimes it takes a few days for me to reply to posts. My email address is on the “About” page. If you are looking for my professional services, please send an email. Thanks.
hi
Please help!
how can i put restrictions on a list box with following conditions on selected options in adobe acrobat x1 professional fill able form? Choose at least 2 options,with a maximum of 4 options, from a list option??
thanks
Hi,
I want to automate a coloring (highlighting) process, please advise how to do it.
Here is the scenario – I have say 50 pdf files and in each file I have to open and use highlighter to specific areas. Lets say, every file has Name and address at fix place which I want to highlight using highlighter. Can I do a automation on this such that without opening the file this properties can be applied? Otherwise if file has to be open, can I copy this highlighter properties from 1st file and apply to remaining 49 files, so that other can also be colored?
Please guide.
Thanks,
Mukesh Patil.
The easiest way to copy annotation data from one document to another document is by using the “replace pages” function: Load your first document (the one that you will use the annotations from), then select to replace the pages of this document, and select your 2nd document as the source for the replaced pages (that’s the document that does not yet have the annotations applied). Save the resulting file under a new filename.
If the highlight annotation is always in the same location, you can use JavaScript to apply it. I would analyze the annotations in a field that you’ve highlighted, and then save that information in a JavaScript data structure, and then – using e.g. a menu item – apply the same highlight information again. You will have to understand the annotation object in Acrobat’s JavaScript API documentation fairly well in order to do that.
I can create small uncomplicated pdf forms but do not know anything about scripting. One of the managers recently asked me if it was possible to update a form I created for them that would have one field locked (i.e. unavailable for text entry) unless the previous text field had been filled in. The field that must be filled is titled ‘Primary Duties’ and the field that should remain locked or unavailable for text entry unless Primary Duties have been entered is titled ‘Rationale’.
After reading this blog I believe if this is feasible the answer will be available here. Any help with this would be much appreciated.
Hi Karl
I am new to scripting and being of elderly age I find it difficult to get my head around validations and calculations. However I have created a number of order forms some of which are quite complex and much to my surprise they work. I now need to create a form that depends on a future date/time and I simply can’t take in how a date can be used.
I have a column of text boxes “Quantity1” “Quantity2” etc, then a column of price boxes which I have labelled “EB1” “EB2” etc which show a price each, followed by a column “LB1” “LB2” etc, which shows a different price each and then a column of “Subtotal” boxes. All formatted as numbers. there is one other box that displays “ToDay” as DD/MM/YYYY .
What I want to do is multiply “quantity1” X “EB1” = “Subtotal1” until the 12/02/2016 and when the time reaches midnight the calculation becomes “quantity1” x the value of “LB1” = “Subtotal1”. I need to show the different prices on the form to show the Early Bird is getting a cheaper price. I could have hidden price each boxes that change value on date change but how is the scripted?
Eric.
Eric, you can use something like this as the custom calculation script in your subtotal fields:
Hugo, use the following script as a custom validation script for the field “Primary Duties”:
Then set the default state for the “Rationale” field to “hidden”. Now when you fill in data, the Rationale field will be become available, if it’s empty, it gets hidden.
Can any one Help me with Acrobat Pdfs,i have 4 pages in pdf.when i want to print,the print option from file menu should not work.when i click on file menu print button pdf should open one new pdf page with back button.
Suresh, I am sorry, but what you want to do is very likely not possible.
Thanks Karl,
1)what if i have 5pages and page 5 with back button,and can i make page 5 to hide and make visible when i click Print option from File menu and only page 5 should visible.
2)Print option should work when i click print button only from my form.
Currently i am working on Acrobat Pro,will it be possible if i choose Acrobat Life Cycle?
Hi Karl,
I’m new to acrobat and Java script.
I’m creating a PDF form using an acrobat Pro DC. One of the fields type that I used is a “Drop Down List”. Is there a way to add more than 20 list, like copy and paste instead of adding the list individually to save my time. or what’s the quickest way to add more than 20 lists.
Thank You for your time.
Zef
Zef, you can set the options of a dropdown control via JavaScript: http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_API_AcroJS%2FJavaScript_API.htm%23TOC_setItemsbc-704&rhtocid=_6_1_8_57_18
Hello,
I have created a form and there are three boxes that i want the person completing the form to enter text into. I don’t want them to be able to save the form without writing something in. Please can you tell me how to do this?
So it can’t be left blank.
Thanks
Laura, you cannot prevent somebody from saving a file – regardless of how much or how little they’ve filled into the form fields. That’s actually a good thing: For a more complex form, you may not be able to finish the form in one session, so the ability to save the partially filled form and to pick it up at a later date is a very good feature to have in a form. Or, when you have a long form to fill out, and you don’t trust your computer, you save along the way. Again, a pretty good feature to have.
So, saving cannot be prevented, but what you can do is to check the form and display a message that says “You are not done yet. Don’t even think about sending this form in the way it is. It will be shredded and burnt and buried unless you fill in the missing information. So please be nice and continue to fill out the form until you have provided all the information required. Thank you for your cooperation.” – or something like that 🙂 This is done using the “WillSave” action script. It is up to you to validate that all information that you require is provided at that point. If you find anything missing, you would display the alert box. But again, this function is called “WillSave”, so Acrobat (or Reader) will save the document, regardless of what you do in that callback.
Can you tell me if such a thing is possible? I have a questionnaire created in MS Word because I want to be able to personalize it to each person I give it to and using the Bookmark/Cross reference tools in word seems to work better than form fields in a PDF. Once that personalization is done, I convert it to a PDF. I want the PDF to have fillable forms but Acrobat finds some of the fields and not others, etc. Is there a way to create, for lack of a better term, a template for telling Acrobat where to place form fields on a new document? I can’t just use a PDF template because each new PDF will have different names but where they need to provide answers is always the same. Is there any way to do such a thing? Thanks for your time.
Chris, you can store all your form fields in a PDF file (Let’s call this “field_template.pdf”). When you want to change the actual form, you create a new file named content.pdf. Now open up the file field_template.pdf and select to “Replace Pages”, and then select the pages from content.pdf to replace all pages on your current file, it will keep the form fields, and will only replace the static content “behind” the fields. This way, you can setup your fields once, and then just swap out the content with one simple operation.
Can anyone help me change the field box color?
I want to change the field color to reflect the Text.
“LOW” = Green
“MODERATE” = Light Green
“SUBSTANTIAL” = Yellow
“HIGH” = Light Red
“VERY HIGH” = Red
var v1 = this.getField(“01C_ScoreRow2”).value;
if (v1 = 10 && v1 = 50 && v1 = 200 && v1 = 400) {
event.value = “VERY HIGH”;
}
if (isNaN(v1) || v1 === “”) {
event.value = “”;
}
Wesley, you may want to read up on correct JavaScript syntax. The comparison operator is “==” and not just “=” as you use it in your “if” statement. Also, the variable “v1” cannot be set to 10, 50, 200 or 400 at the same time (which is what you are testing for with the “&&” operators), I don’t know your exact requirements, but I assume you want to use the or operator “||”.
As to how to set colors, this tutorial has the information you are looking for: https://acrobatusers.com/tutorials/using-colors-acrobat-javascript
Sorry Karl,
I don’t know why it pasted in that format, but that v1 script works.
I need help after it converts to Low, Moderate, Substantial, High and Very High.
“LOW” will = Green background
“MODERATE”will = Light Green background
“SUBSTANTIAL” will = Yellow background
“HIGH” will = Light Red background
“VERY HIGH” will = Red background
var v1 = this.getField(“01C_ScoreRow2”).value;
if (v1 = 10 && v1 = 50 && v1 = 200 && v1 = 400) {
event.value = “VERY HIGH”;
}
if (isNaN(v1) || v1 === “”) {
event.value = “”;
}
Wesley, the way the script shows up here, it cannot work. Assuming that any value above 400 will be considered “very high”, anything above 200 “high”, and so on, I would use something like this:
var v1 = this.getField("01C_ScoreRow2").value;
if (v1 >= 400) {
event.value = "VERY HIGH";
event.target.fillColor = event.target.strokeColor = color.red;
} else if (v1 >= 200) {
event.value = "HIGH";
event.target.fillColor = event.target.strokeColor = color.red;
} else if (isNaN(v1) || v1 === "") {
event.value = "";
event.target.fillColor = event.target.strokeColor = color.transparent;
}
else {
event.target.fillColor = event.target.strokeColor = color.transparent;
}
I did not cover all the cases, only the two that result in the red background, and the default case with a transparent background. You will have to set both the “strokeColor” and the “fillColor” (which is the actual background color) because when you have “Highlight Existing Fields” selected, you won’t see the background color.
Awesome, Thank You Karl!
Does anyone know of a way to force a number to be shown, regardless of what is entered? I’d like to enter a discount into a form but the discount is a fixed number. I’m already using the following Format custom script
if (event.value == 0)
event.value = “”;
else
event.value = “$” + event.value;
and the following Validate Custom script
if (+event.value>0) event.value=-event.value;
Any thoughts on forcing the output to be a specific number regardless of what the user enters?
Thanks for your help.
Clee, you can certainly do that using either a custom format or a custom validation script. What exact behavior are you looking for?
Okay, so I have several fields. They are all numbers and use either user input, or math equations based on the user input.
Field A is user input.
Field B is user input.
Field C is = to Field A if A is B.
Field D is = Field C + E, F, & G.
Fields E, F, & G are math equations.
Field C changes just fine if Field A or B is changed. Works great.
Field D however, does not always recalculate itself when Field C changes as a result of Field A or B. It will often keep showing the previous (correct) answer from before Field A or B changed, as if it does not detect the new information.
How can I force Field D to validate itself every X seconds? Or force it to detect changes in Field A & B so that it knows to recalculate?
Well… some of that got edited out.
Field C is = to Field A if A is less than or equal to B. It is equal to Field B if A is greater than B.
James, when you have a calculation script, it gets automatically called whenever something in the form changes. There is no need to validate something every x seconds – that would be just wasting CPU cycles. You have to make sure that your calculations are being performed in the correct order. There is an interface to set the calculation order, but my preferred way of doing this is to have only one field to trigger a calculation script. This way, you don’t run into any accidental problems with wrong calculation sequences. Ideally, you would create a document level function that you then call from one field. That function would perform all calculations in your form.
Hi Karl,
I need ANY entered number to reflect a specific number [55 in this case]. So if the user enters 60, 55 will show up regardless. Also, the 55 is part of a calculation in another cell so it needs to reflect 55 and not their entered number so the calculation works out correctly.
Clee, then please allow my naive question: Why even allow the user to enter a value?
Karl, what would be the interface to check that the calculations are being done in the correct order then? Or is it simply the order of the fields when in form edit mode?
I can’t really combine the fields or have only one calculation as I need them all depending on what I am trying to look up at the moment about the subject.
Thanks.
Ok, nevermind. I found the calculation order. Fixed some things. Now, unfortunately I have another problem.
var a = this.getField(“Modifier”)
var b = this.getField(“Max Bonus”)
if (a.value b.value) {event.value = b.value} else
if (b.value === undefined) {event.value = a.value}
For some reason that I cannot fathom, when the third statement comes into play (a>b) it does the correct math, but changes the value of the Modifier field instead of just putting the proper number in the current field! Anyone know a way to stop this? Because as far as I can tell this code should only affect *this* field, “field C” by using data that is *referenced* in Field A. It should never *alter* field A.
Gah. the greater than and less than signs messed up again…
var a = this.getField(“Modifier”)
var b = this.getField(“Max Bonus”)
if (a.value -less than sign- b.value) {event.value = a.value} else
if (a.value = b.value) {event.value = a.value} else
if (a.value -greater than sign- b.value) {event.value = b.value} else
if (b.value === undefined) {event.value = a.value}
For some reason that I cannot fathom, when the third statement comes into play (a is less than b) it does the correct math, but it also changes the value of the Field A instead of just putting the proper number in the current field!
Anyone know a way to stop this? Because as far as I can tell this code should only affect *this* field, “Field C” by using data that is *referenced* in Field A. It should never *alter* field A.
It also seems to not work if there is a zero in field B.
Nevermind, got it figured out. Thanks for the help.
Karl,
As silly as it sounds, we’ve found that offering a discount and having the user put the discount amount in, nets us a higher early-bird return. The rub is that people try to be coy or simply erroneous in their entry. Having the ability to offer the user the ‘money saving experience’ but facilitate our back-end use in a correct tally satisfies both ends.
Clee, I still don’t understand: You are allowing the user to input a number, but then override it with something different.
Yes Karl as silly as this seems. The discount can only be one number, but sometimes people put in a different number [either to see if they can beat the system and pay less or because of a typo]. We need the number to always be 55.
In this case, I would use the following as the custom validation script:
if (event.value != "") {
event.value = 55;
}
That worked, thanks. Is it possible to add other specific numbers to the ‘approved’ list? Like 25 and 15?
Hi , I am new to fallible PDF and java Script writing, I need help in getting 14% vat from the Sub total amount, i have tried:
in the simplified field notation: Sub/Total*0.14, first try to see if it works it gave me a 1 answer and then stayed 1, now its just blank. I need this form done very urgently.
Can anyone please help me, in showing me the correct way of writing the script.
Sonja, what exactly is your “sub total” field name? In your simplified field notation calculation, it looks like you are using two fields: “Sub” and “Total”, and dividing them before multiplying by 0.14 – that does not look correct. Let’s assume you have a field named “SubTotal”, then in the simplified field notation, the VAT would be calculated like this:
SubTotal * 0.14
Hi!
I found your page via a web search and hope you can help with something.
I’m building a proposal form in PDF and have a page that breaks out the various options at different price intervals. For each field I have a variation of the below code:
var plan = Number(this.getField(“OPPAOption1”).value);
if (plan==100) event.value = “$100”;
else if (plan==125) event.value = “$125”;
else if (plan==150) event.value = “$150”;
else if (plan==200) event.value = “$200”;
else event.value = “”;
As you can see, the script looks at the value in the field “OPPAOption1” and returns a pre-determined value based on one of four possible variables entered; 100, 125, 150 & 200.
My question is whether or not it is possible to alter this code so that if one of the four variables is not entered then the field will allow manual entry of data?
For example, if I enter “115” into field “OPPAOption1” I want to be able to then manually enter the data I want.
Is that possible?
Thanks
Keith, I assume you are using the script you provided as a custom calculation script. You can modify the script so that it changes the “readonly” property of ht field based on what was entered in “OPPAOption1”:
Karl,
Hoping you can help. I have a few fields that are being calculated but need to have a minimum charge and the simple validation isn’t helping — total newbie to Javascript so I need help with a custom validation script.
Field A – Qty
B/C/D – Dimensions
E – Cubic footage
F – total cubic footage (A*E)
G – Monthly rate —> This is cubic footage * $0.32, OR $15, whichever is greater.
My calculations are all good, but as far as the $15 minimum charge, I can’t: a) override the calculated rate manually, even with the cell unlocked or b) figure out how to auto-populate $15 as the monthly rate after the calculations have run without $15 populating throughout the entire form. The basic validation I’ve tried to use to set a $15 minimum value (without actual scripts) pops up dialog boxes for all rows before the data gets entered. Hopefully that makes sense — would love your help and to learn a bit more about how this works!
I actually figured it out thanks to a post you made on answers.acrobatusers.com!
Thanks 🙂
Audrey, glad that I could help, even if it was not here on my own site 🙂
Hi Karl.
I thought I provided a response. My apologies for the delay.
Your code worked like a charm.
Thank you very much!
Hi Karl.
I’ve tried figuring this out on my own but I’m stumped.
Everything works fine with one tiny hiccup. If I manually enter data into the field, that data is deleted when I tab out.
Quick recap: I have a form consisting of 4 columns of fields, 32 fields per column. The uppermost column is where either one of four (4) predetermined variables will be input OR a custom variable will be input. The four predetermined values are:
100
125
150
200
If any one of those values are placed in the uppermost field then all fields in the column below should show a set value and flip to read-only. If a custom variable is entered then the fields should switch to editable mode so data can be manually entered.
The script being used is:
var ro = true;
var plan = Number(this.getField(“OPPAOption1”).value);
if (plan==100)
event.value = “$100”;
else if (plan==125)
event.value = “$125”;
else if (plan==150)
event.value = “$150”;
else if (plan==200)
event.value = “$200”;
else {
event.value = “”;
ro = false;
}
event.target.readonly = ro;
The issue I’m having is if I enter a custom variable in the top field, say 75, the fields lose their read-only status and I can manually enter data into the fields below but upon tabbing out of that field the data is wiped.
Any thoughts?
I can send you the file if you wish.
Thanks.
Hi Karl,
I’ve been trying to figure out how to write the script for a form, but I don’t know enough about acrobat. I hope that you will help me.
I have four text fields. “BoxSize”, “TaxCode”, “Price” & “Tax”
BoxSize is filled with numbers 0-9 & TaxCode 1-2 based on the numbers entered on these tow fields, “Price” & “Tax” would automatically populate
Example
If BoxSize is 1 & TaxCode is 1, then Price would populate $50 & Tax $2.36
If BoxSize is 1 & TaxCode is 2, then Price would populate $50 & Tax $2.08
If BoxSize is 3 & TaxCode is 1, then Price would populate $65 & Tax $3.06
If BoxSize is 3 & TaxCode is 2, then Price would populate $65 & Tax $2.71
Thank you in advance for any help!
Yoshi
Yoshi, you may want to do yourself a favor and get a good introduction to JavaScript. Your problem has (almost) nothing to do with Acrobat, and is about using control structures in JavaScript. What you need is an if/else statement. Something like this will work:
Hi Karl,
I’m using some of your code provided in earlier comments in a validation script, and it does exactly what I need. The issue I’m running into is I have a button on the form that on Mouse Down runs the Reset a Form action. When I click this button, the script is throwing the app.alert message for each (validated) field that has data entered in it. The code I am using is below. Do you have any suggestions? Thanks!
if (event.value != “”)
var alphaNumericPatt = /^[0-9]*$/;
event.rc = true;
if (event.value.length ==4 && alphaNumericPatt.test(event.value)) { event.target.textColor = color.black; } else { app.alert(“Time must be entered in 24hr (military) format and not include a ‘:’. Ex. 0600, 1330”); event.value = “”; }
Chris, I think you need a pair of brackets to make this work:
When you reset the form, the field’s value is very likely an empty string (if it’s not, then test for the default value instead of the empty string), so we need to make sure that the empty string goes through validation without a problem: With the modified code, the whole block gets skipped when the field is blank.
Perfect! Thanks, Karl!
Hi karl
I’m new in Js plz help me
Im creating form which is having three textbox(1,2,3) texbox1 like that (MHR-10100-1-0)having mix alphnumirical with dash(-) i want to show textbox2 only first three alphbites from textbox1 having, and textbox3 show only middel 5 numbers
Hi,
Hoping you can help me out with this. I am creating a sheet with six months of reimbursement of 50% of the monthly cost, up to $50; one line for each month. In each line, I created a field for cost input and then a second field which calculated 50% of cost input and then verifies that the result is under $50. I have the basic code figured out, but when the amount is ignored and the user moves to the next month and enters another amount for which the reimbursement would be over $50, the user now gets two warnings, as the initial warning from the first line repeats. I want to add an option to “Hide this message” in my pop up box. Please advise and thank you in advance for your assistance!
event.rc = true;
if (event.value && (Number(event.value)50)) {
app.alert({cMsg:”Reimbursement limited to $50 maximum.”, nIcon:1, oCheckbox:oCk})
hideWarning1 = oCk.bAfterValue;
}
rajsam, you can use regular expressions to extract that information. Take a look at the following code:
This establishes a regular expression with 4 groups (the first one only capital letters, the others groups of digits) separated by dashes. We get the first group and assign it to the first field via a custom calculation script. When you use the same script for the second field, but change the [1] to [2] in the line ‘event.value = p[1]’, you can assign the second group to that field.
Take a look at “example3” in the documentation for app.alert(), it shows how you can suppress a dialog: http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_API_AcroJS%2Fapp_methods.htm%23TOC_alertbc-4&rhtocid=_6_1_8_6_1_3
Hey Karl,
I have a form that will require users to verify that all the data has been filled in. The fields are required, but I do not want them to have the red outline. Also, I have put a button on the form called “Verify Data”. This button needs to highlight all empty fields with a red border (hence why I don’t want the red border for required fields), but then, if all fields are filled in, to populate a two digital signature blocks for users to sign. I have no clue how to javascript this, so if you could help, I’d greatly appreciate it. Thank you
John,
you can do that by adding your own “validation” routine that will go through all fields, and then identify those that are not yet filled in, and mark with them with a red border, and remove any red borders from all other fields. Unfortunately, such a solution is a bit more complex than what I can do here in this blog. If you are interested in my professional consulting services, please feel free to get in touch with me by email. My email address is on my “About” page: http://khkonsulting.com/about
Hi Karl,
I’m trying to make a form which auto-populate the same field in other pages. I have tried naming the fields with same field name.
But what I want is, whenever the first field is filled-out, the other fields with the same name, will also be auto-populated and at the same-time make it read-only. But the first field is not read-only, only the fields on the other pages with same field name.
How to do this using javascript?
TIA
H Karl,
I have an adobe file where I need to have the fill color of the text box change according to what is entered in the field. For example, if the entry is 1 the color is red, if the entry is 2 the color is yellow and if the entry is 3 the color green. I have no java experience so I need help. Thanks!
Hi Karl,
Is there a way to create an error message for form field “Last 4 digits of SSN” saying, “The entered value needs to be a number”? I’ve tried the arbitrary mask, on the format tab, but I can’t edit the error message.
Any assistance would be helpful.
Thank you.
Hi I am using Adobe Pro for fillable order form. we have a special price the first item for $11 and any additional will be for $9
Could you please help with the formula.
Thanks,
Deirdre, take a look at this tutorial, it demonstrates how to use colors in a PDF form (and change the color of a field based on it’s value): https://acrobatusers.com/tutorials/using-colors-acrobat-javascript
Steven, you can do this with a custom validation script. To test if something is a number you can run the reverse test to see if it’s “not a number” (or NaN) and then just negate the output. See here for more information about isNaN(): https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/isNaN
Maha, what you are asking for is well outside of the scope of this blog post. You can always hire me to do the work for you, but if you are interested in free help, please post your question on the Adobe forums: https://forums.adobe.com/community/acrobat/acrobat_scripting
Reymund, this is not possible by using the same filename – the fields with the same name will share – besides the data – also the read-only state , so if one field can be modified, all fields will be writable. What you can do is name the fields differently, and then use a custom calculation script in the read-only field to copy the value from the writable field:
Hi Karl great blog and tutorials.
I understand a little of Javascript and am working with an Acrobat file to create some function. For instance -> 4 radio buttons (value 1-4). Depending on what button is selected will determine the choices for the next drop down menu..
Example: if radio button #3 is selected, list of dropdown items is only 3a, 3b, 3c.
Example if radio button #1 is selected, list of dropdown items contains only 1a, 1b, 1c.
What is an example of the code needed for this?
John, Take a look at this AcrobatUsers.com question: https://answers.acrobatusers.com/How-do-I-get-use-script-to-use-different-drop-down-lists-q284851.aspx
Thx Karl! I’ll look into that.
Hi Karl,
I am working with Abobe Acrobat DC interactive form, I have a drop menu with 70+ entries, after reading your earlier posts I understood that only text fields can be made mandatory fields by selecting “Required”.
Here is my query, I need to make it mandatory field (the drop-down menu which is called “Store Name” e.g: 201 Hawthorn, 202 Toorak, 401 Townsville), I also have a “Submit a form button via email”. How do I force this validation where user must select a store name and cannot email this PDF?
Your help is highly appreciated.
Regards
Sumit Gabbi
Sumit, you would have to create your own validation function that you call before you actually submit the form. In order to prevent the email submission when validation fails, you cannot use the standard “Submit a form” button, instead, you will have to use the equivalent JavaScript function Doc.submitForm(): http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_API_AcroJS%2FDoc_methods.htm%23TOC_submitFormbc-106&rhtocid=_6_1_8_23_1_105
You would validate first, and then, based on your validation results, either call Doc.submitForm(), or display an error message.
Karl,
Thank you for your help.. It worked for me..
I have another query as well, Is it possible to submit an Acrobat form via OWA?
We no longer use any thick client, if not, are there any options that I can look at?
Regards
Sumit
Sumit, Adobe Acrobat supports a Yahoo and Google email directly, but not OWA. If you can configure it via IMAP/SMTP servers, it should work, if that’s not an option,you may have to save the file and then attach it manually to the email.
I’m trying to set a custom script to validate a field called Client account Information.
which the user should input ( company name address city state and zip ) . this field is often left blank, I want form to not print unless the field in field out .
Mondo, you will need to create a “document will print” document action that first of all informs the user that something is missing, and then marks up the document (e.g. with an overlay of a large form field that contains the message “Information is missing – please correct and then print again”). There is nothing you can do to prevent printing, all you can do is make sure that it’s clear that the printout has missing data (e.g. with that large message).
Karl Heinz Kremer,
My job is to transfer clients data in to a pdf form. I am trying to move the date and month of client’s birthday to other box and leave the year in the original box. i have to move the date and month every time and i am trying to find a way so that it does it itself
For example:
client’s brithday is dd/mm/yyyy (01/02/2016) it always come with date month than year in the order
This is what i am trying to do”
01/02/2016 and move “01” to the date box and move “02” to the month box
Marco, you can certainly do that. There are different ways you can approach this problem. You can for example use regular expressions to disassemble the complete date string and extract the year, month and day and then assign the appropriate data to the individual fields. If you need professional help with this, feel free to contact me via email. My email address is on the “About” page.
Hi Karl Heinz!
First of all, thank you for your post. It’s very helpful.
Second, I must apologize for my poor english.
And third, my question.
I’m programming a JavaScript that creates 3 text fields with the same name (because they must share his value), but with different properties (font type, color and page). The format of data is 999/9999, where first 999 block refers to a order number provided by the client, and second 9999 block refers to current year.
I have not problem programming the data check (asked at the moment of run the script ) to be correct, but I need to set a permanent arbitrary mask on the first field. The client send me new order numbers that affect this file, I must change the 999 value and print the renewed file (I can have 70 different files and counting, waiting new orders numbers).
At this moment, I have a PDF with the tree fields, created by hand, and I copy and paste they at right page. Then, I enter the value on the first field to change the rest. Actually, I use a arbitrary mask (999/9999) to check that the value is ok.
I wish to know if I can put this validation mask embebed in the field, needing not more JavaScript running in the dark to work properly next time I must change values, right like now, with hand made fields.
I hope my problem is clearly exposed, but I’m not very sure, sorry.
Thanks in advance.
I was looking for the field property that can storage this kind of validation, because I belive that all the field properties showed when you create a field in Acrobat can be accessed via JavaScript, but I don’t find anything.
Jose, unfortunately, I don’t understand what you are trying to accomplish.
Hi Karl!
Sorry, too long explanation with a bad english = I DONT UNDERSTAND YOU 🙁
Finally, i can do it adding a script ( the validation code) at doc level with addScript:
this.addScript(“validarCampo()”,”event.rc = true;fechaCompleta = new Date();anio = fechaCompleta.getFullYear();patronValidacion = \”OI: \\d\\d\\d\\/\” + anio;var miRegExp = new RegExp(patronValidacion);if ( !miRegExp.test(event.value) ){app.alert(\”Revísalo, anda…\”);event.rc = false;}”);
and inserting it in the field with:
this.getField(“Texto1”).setAction(“Validate”,”validarCampo();”);
I needed to add a validation script to a form field VIA Javascript, not manually like you do in your example. I must run a script that create the fields, add the initial values and validation code, and wait to new values to be entered and validated. This method works fine.
Im a book printer. Only one of my clients need to put a secuencial number EVERY TIME he prints one of their books. The first book he prints at january 2016 needs to display “OI: 001/2016”, the second “OI: 002/2016” and so on. We are not his only printer, so we cant automate totally the numeration (with a database).
But we can automate a lot with this script.
I hope you can understand me better now.
If not, thanks a lot for your attention, and never mind.
Greetings from Spain
Jose
Hi Karl
i am building form where there is one field there should accept the value of HC at the beginning and then 8 digits . can you please help me to do so?
thanks
Mohamed, this can be done with a regular expression. Look up how regular expressions work in JavaScript (this is not specific to Acrobat, any resource about JavaScript should have some information about RegEx handling).
Hi there, I’m a little new to Acrobat I’m trying to achieve a calculation in my PDF but failing miserably below is what I’m wanting to achieve please help before I give up!
I have three fields, two of which are visible and one is hidden:
lbs – (this is visible and requires user input)
kgs – (this is visible and should be the result box)
calculation – (this is hidden, and the value is 2.2)
In other words I want the user to insert their lbs (in weight) and for the conversion to kg to be displayed in the kgs box so (user input / calculation (2.2) = kgs
please help me, what do I need to do and where please?
Kind Regards
Marie, you need to add information to the “Calculate” tab on the field’s properties dialog. You can either use the simple field notation and enter something like this (assuming that the field name for the user input field is “lbs”):
lbs / 2.2
There is no need to save the “2.2” in a separate field.
Hi Karl,
I’m trying to validate data input in a text field based on data stored in another field and was wondering if you had any magic to do this.
For example:
Field 1, which is locked and hidden on the form contains a value of “NP131415”
Field 2, requires input and should only accept the value of “NP131415”
Is it also possible for the data input in Field 2 to only accept the last 4 characters of field 1.
Many thanks,
Nigel
Nigel, you can certainly do that – you can use all JavaScript string methods in Acrobat’s JavaScript, so one option would be to get the last four characters of field 1 by using something like this:
var str = this.getField("Field1").value;
var lastFour = str.substr(str.length - 4);
console.println(lastFour);
Now you can use this substring in your comparison.
Dear all ,
My form has 4 fields (“Price1” – “Preis4”) with numbers (prices). Is there any javascript, with which I can identify the lowest price (automatically highlighted in green color and the highest in red color?
In addition the script should populate the lowest vaue in field “MIN1”, the average value in field “AV1” and the highest field value in field “MAX1”.
For all 4 price fields (“Price1” – “Preis4”) I set calculation script as follows:
var NamesArr = [“Price1”, “Price2”, “Price3”, “Price4”];
for (var i = 0; i < NamesArr.length; i++) this.getField(NamesArr[i]).setAction("OnBlur", "calcFields();");
For document javascript I set following script:
function calcFields() {
//make an array of the numbers and the field names that correspond with them
var valArray = [[1],[2],[3],[4]];
var total = 0;
var NamesArr = ["Price1", "Price2", "Price3", "Price4"];
for (var i = 0; i < NamesArr.length; i++) {
var Nm = NamesArr[i], fld = this.getField(Nm);
fld.textColor = color.black; //reset all the field colours
if (fld.value && !isNaN(fld.value)) {
valArray[0].push(fld.value);
total += fld.value;
valArray[1].push(Nm);
}
}
//get the max value and make it red
var maxVal = Math.max.apply(null, valArray[0]);
var maxIndex = valArray[0].indexOf(maxVal);
var maxFld = this.getField(valArray[1][maxIndex]);
if (maxFld) maxFld.textColor = color.red;
this.getField("MAX1").value = maxVal;
//get the min value and make it green
var minVal = Math.min.apply(null, valArray[0]);
var minIndex = valArray[0].indexOf(minVal);
var minFld = this.getField(valArray[1][minIndex]);
if (minFld) minFld.textColor = color.green;
this.getField("MIN1").value = minVal;
//get the average value
var avgVal = total / valArray[0].length;
this.getField("AV1").value = avgVal;
};
The validation is working, however not working for comparison of ZERO numbers for example: 0,1; 0,3; 0,45, 0,6;
In this the ithe MIN value is shown in the right way (in green color), however the MAX value is not shown in red color and also in the field "MAX1" only value=1.
I would be grateful for any idea or comments on problem solution.
BR
Florian
Florian, I assume this is the same problem you are trying to resolve on Adobe’s forums. Do you still have problems? If so, I would recommend sticking with the Adobe forums (where I participate as much as my time allows). Your problem is not directly related to the blog post you commented on, so Adobe’s forums are the more appropriate place.
Hi Karl,
This has been driving me nuts for a couple of days. I have a dropdown box with Yes and No as responses as to whether or not the user is tax exempt. If Yes, tax will only be charged on the shipping cost, if no, it will be charged on the sum of the subtotal cost and the shipping cost. I have been able to write several scripts that were accepted, but none of them will run and I have no idea why. Here are a couple:
var TaxExempt=this.getField(“TaxEx”).value;
if (TaxExempt !=”Yes”){ event.value=(ShipCost*0.0825);} else {event.value=((ShipCost+SubtotalCost)*0.0825);}
var TaxTotal=Number(this.getField(“SubtotalCost”).value+this.getField(“ShipCost”).value);
var TaxEx = this.getField(“TaxEx”).value;
if(TaxEx==”Yes”){event.value=ShipCost*0.0825;}
else {event.value=((SubtotalCost+ShipCost)*0.0825);}
Any help would be much appreciated! Thank you.
Hi Karl,
What a resource you are! Reading through I almost found my answer. I need to have a script the forces 4 numeric and 1 alpha. example “1234A”
I got the 4 numeric to work from a previous post. But I could not get the 1 apha character to work.
Thank you
Gary, in this case, it’s easier to use one of the standard format options. Select the “Format” tab, then select to use a “Special” format category and pick “Arbitrary Mask” from the list. In the input field type 9999A – this will allow four digits followed by a letter.
Siobhan, it looks like there are a few lines missing in either script. Try this:
var SubtotalCost = Number(this.getField("SubtotalCost").value);
var ShipCost = Number(this.getField("ShipCost").value);
var TaxEx = this.getField("TaxEx").value;
if (TaxEx == "Yes") {
event.value = ShipCost * 0.0825;
} else {
event.value = ((SubtotalCost + ShipCost) * 0.0825);
}
Thank you so much, Karl! I just had to remove the extra ” and it worked perfectly!
This is an amazing resource that you provide, and it is much appreciated.
Thank you again.
Siobhan, I am sorry about that extra quote. I removed it from my comment. That’s the problem when you type code in a blog comment, there is no syntax checker that tells you that that program will never run 🙂 I am glad that you found the problem.
I am trying to compare fields. So if one field is filled in, the other must be and vice versa. I have setup in a button such that the field that needs to be filled gets turned red, and I set the focus to the field. The problem is… unless I click outside the culprit field, it still says the error exists… so for example the user just types a number into the field to fix the problem, but never presses enter or clicks on a field in the form… when the script runs behind the button, it errors out thinking nothing has been done.. but clicking it a second time it realizes the problem is fixed…
if (getField(“Proposed TIME DEP Z2″).value!=”” && getField(“OAT2″).value==””){
app.alert(“Please fill out the following fields: OAT2”,3);
this.getField(“OAT2”).setFocus();
this.getField(“OAT2”).fillColor = color.red;
}
else {
this.getField(“OAT2”).fillColor = color.transparent; }
Chris, you can trigger your script (e.g. wrapped into a function) from a custom keystroke event on the “Format” tab. For this to be available, you have to select a “Custom” format.
Hi Karl
I wonder if you can help. I am trying to validate a field which is a registration number issued by one of several governmental bodies. I need to check the format is in either the format 999999 or 99A9999A.
I tried adapting one of he scripts you suggested earlier in the thread but to no avail.
Any assistance would be greatly appreciated.
Terry, you need a custom validation script that uses “regular expressions” to test the two different patterns. Try the following:
// allow either 999999 or 99A9999A
var re1 = /^\d{2}[A-Za-z]\d{4}[A-Za-z]$/;
var re2 = /^\d{6}$/;
event.rc = true;
if (!re1.test(event.value) && !re2.test(event.value))
{
app.alert("The entered value needs to be in the format of '999999' or '99A9999A'!");
event.rc = false;
}
Thanks Karl, this works well, however I have just realised that I also need to allow the possibility of a blank entry as otherwise I cannot save the form without a valid entry in the field. I also need a null entry available if the person is not or not yet registered and therefore does not have a number at all yet.
Thanks for all your excellent help.
Terry,
you need to add a check for an empty string as well.
You could change this one line:
if (event.value != "" && !re1.test(event.value) && !re2.test(event.value))
Thanks Karl, that works perfectly. Your service here is a great help to us lesser mortals.
Hi,
I am trying to enter a date field into my form, and set a rule that the date entered has to be before a May 16, 2017. Unfortunately on the text field properties box, I cannot select the “Field value is in range” tab, but I can select “run a custom validation script”…
I am not well versed in writing java script.
Lauren, you need a custom validation script that checks that the date is within a given range. Here is a tutorial about working with dates and times in Acrobat’s JavaScript: https://acrobatusers.com/tutorials/working-with-date-and-time-in-acrobat-javascript
Hi Karl, I am new to coding and have a form that needs these fields:
qty unit price case price subtotal
the subtotal box would be an “if/then” scenario
the customer wouldn’t order a single unit if they they are ordering a case, and vice versa–
so I want the form to calculate either the qty x unit price or qty x case price,
then on top of that if they order 3 cases they get one free.
thanks for any help you can offer.
Maria, what you want to do has nothing to do with validation (which is the subject of the post you commented on). You need a calculation script that can take in information from different sources (the fields, information about case vs. single item and discount) and then comes up with the correct subtotal/total. I can certainly help you, but that would require my consulting services. If you are interested, please send me an email. My contact information is on the “About” page.
Hey Karl.
The Submit button on my form is set to validate two calculated fields that need to be equal. If they are not equal an popup message informs the user of the error. I’d also like it to change the text or highlight color of the calculated fields in order to call attention to them. I can’t figure out how to do this. Can you help? The code I’ve got so far is based on your suggestions and only alters the color of the Submit button.
Any help you could provide would be greatly appreciated!
var A = this.getField(“Total Receipts”).value;
var B = this.getField(“Grand Total Paid”).value;
var Receipts = A.value
var Paid = B.value
event.rc = true;
if (A != B)
{
app.alert(“The Total Receipts and Total Paid fields must be equal. Please check for errors (CURRENCY + COIN + CHECKS + CHARGE = SITTING FEE + PROOF DEP. + ORD. PAYMENT).”);
event.target.textColor = color.red;
}
else
{
event.target.textColor = color.black;
}
Wouldn’t you know it, as soon as I submitted my question I was able to solve the problem myself! I also added the actual email submit instruction. It seems to work perfectly.
One additional question, however: Is it possible append the file name of the submitted form with the value from the date field? I’d like it to look something like “Daily Cash Sheet 03/04/17.”
var A = this.getField(“Total Receipts”).value;
var B = this.getField(“Grand Total Paid”).value;
var Receipts = A.value
var Paid = B.value
event.rc = true;
if (A != B)
{
app.alert(“The Total Receipts and Total Paid fields must be equal. Please check for errors (CURRENCY + COIN + CHECKS + CHARGE = SITTING FEE + PROOF DEP. + ORD. PAYMENT).”);
this.getField(“Total Receipts”).fillColor = color.yellow ;
this.getField(“Grand Total Paid”).fillColor = color.yellow ;
}
else
{
this.getField(“Total Receipts”).fillColor = color.transparent ;
this.getField(“Grand Total Paid”).fillColor = color.transparent ;
this.submitForm(“mailto:emailaddress.com”);
}
Jeff, unless you want to write out the file to this different filename, you cannot rename the file during a forms submission. To write the file, you would need to install a folder level script (which means you would need access to every computer that this form is being filled out on). You can learn more about this method here: https://acrobatusers.com/tutorials/how-save-pdf-acrobat-javascript
Hi Karl,
Thanks for the great article.
I’ve had a look through all the comments but couldn’t seem to find the answer so I’m hoping you could help.
So I’ve already set up an OK button at the end of my form which checks the form to ensure that all required fields have been filled out.
What I am hoping to do is either set up another OK button or add code to the existing button code which carries out the process that you described in your blog of checking relevant fields for text color.
Current button code was taken from https://forums.adobe.com/thread/1223607:
var emptyFields = [];
for (var i=0; i0) {
app.alert(“Error! You must fill in the following fields:\n” + emptyFields.join(“\n”));
}
Many thanks,
Tom
Tom, the following script will loop over all fields and check for the
textColor == color.red
condition:This assumes that you already have declared your
emptyFields
array variable. You can of course display the message directly in this loop, but the better way to do this is to collect all field names and then only display one error message, listing all names.Hi Karl,
I have a couple questions for you. So I am trying to create a form for my department. I have a button at the bottom that is coded to do an app.mailmsg. My questions are:
1. What javascript do I need to stop the mail.msg from running until all of my required fields are filled out?
2. When the button with the app.mailmsg is clicked it prompts for sending the email. I would like to send silently. I’ve researched and am pretty I need to use Trusted function but have no idea how to incorporate it into my code.
var cMySubject = this.getField(“Summary_seFNneI6RXkhXEMf4nx-7Q”).value + “\n”;
cMySubject += this.getField(“Summary_seFNneI6RXkhXEMf4nx-7Q”).value + “\n”;
var cMyMsg = this.getField(“First name_qgD22ZwweyQwahoWHjgIRw”).value + (” “) + this.getField(“Last name_m37t-4nFRX17oxloJm1Sag”).value + “\n”;
cMyMsg += this.getField(“Email address_*lZeWetAe*LSSeOW5LTWnA”).value + “\n”;
cMyMsg += this.getField(“Description_f4aoAFZLKSnp-EGpLPFIHw”).value + “\n”;
cMyMsg += “#priority ” + this.getField(“Severity of issue_m5FdjvhmNqi*-QfCEJyiQA”).value + “\n”;
cMyMsg += “#category ” + this.getField(“Problem category_JHcjPWpl6yPlcFnaIfF7fg”).value + “\n”;
app.mailMsg({
bUI: false,
cTo: “user@example.com”,
cSubject: cMySubject,
cMsg: cMyMsg
});
Hi,
I have a form [https://s8.postimg.org/79qm44ucl/acro_form.jpg]. There is a Text Field and two Check Boxes grouped with the Export Values 0 & 1. I’d like to simplified the Check Boxe(s) INFO script:
//———————————————————————-
var nValue=this.getField(“Kwota1”).value;
if (nValue == 0) {
app.alert(“Fill first the Text Field or give it up”);
this.resetForm(“cb1”);
getField(“Kwota1”).setFocus();
}
else { event.value=nValue; }
//———————————————————————-
I’ve made an array
//———————————————————————-
var tFields = new Array (“Kwota1”, “Kwota2”, “Kwota3”, “Kwota4”, “Kwota5”, “Kwota6”, “Kwota7”, “Kwota8”, “Kwota9”, “Kwota10”);
var cFields = new Array (“cb1”, “cb2”, “cb3”, “cb4”, “cb5”, “cb6”, “cb7”, “cb8”, “cb9”, “cb10″);
for (indx = 0; indx < tFields.length; indx++) {
var tValue = this.getField(tFields[indx]).value;
var cValue = this.getField(cFields[indx]).value;
if (tValue == 0) {
app.alert("Fill first the Text Field or give it up”);
this.resetForm(cValue);
getField(tValue).setFocus();
}
}
//———————————————————————-
Unluckily my “simplified” script checks all fields and returns… You know 🙁
There is no really row index, really text field or check box name…
Is it feasible/possible in a simple way?
thanks for any help
Karl,
Thank you for the response, I’m not sure if something is missing from the script you answered with? All that’s showing on my screen is:
“for (var i=0; i”
“This assumes that you already have declared your emptyFields array variable. You can of course display the message directly in this loop, but the better way to do this is to collect all field names and then only display one error message, listing all names.”
Thanks,
Tom
Tom, sorry about that. Something went wrong with the formatting, and it just dropped most of the code that I tried to post. It should be OK now.
Karl,
Thanks for the correction. Unfortunately when I paste the code into the Run a JavaScript section I get a syntax error 1 on Ln2, Col 35:
” var n = this.getNthFieldName(i);”
My JavaScript knowledge is very limited so I’ve tried to look at syntax corrections for this but failed.
Thanks,
Tom
Tom, I am really sorry about this, but my “make the reply show all the code” change introduced a problem in the actual code. The “i+” in the for statement is supposed to be a “i++” – I corrected that.
Karl Heinz,
The simple script you published to select a text string works well in one field but I am trying to setup an ALCOA checklist for a training module and if I copy the script to the next text form field below every time I enter the first value it replicates in the fields below. Obviously I need to rename each field somehow. Help please – I have about 30 different questions and therefore 30 different text fields where students enter their answers.
Here is the script:
event.rc = true;
if (event.value != “” && event.value != “AAAA” && event.value != “BBBB”)
{
app.alert(“The entered value needs to be either ‘AAAA’ or ‘BBBB’!”);
event.target.textColor = color.red;
}
else
{
event.target.textColor = color.black;
}
How do I name each field uniquely and so prevent replication of an entered answer (usually just the letter “A”to “O” as per ALCOA or the phrase “GDI”) in the first field?
many thanks.
Allan T
Allan, when information gets automatically copied to other fields, that is usually (unless you created a custom calculation script that actually copies the data) because the fields share the same name: This is actually a feature of PDF forms – when two or more fields have the same name, information entered into one will automatically show up in the others. You can rename a field by bringing up it’s properties dialog and then – on the “General” tab – change the field “Name”. Another way to do this is to create only one field in your document and set up all the scripts you want, and then right-click on that field and select to “Create Multiple Copies” – this will rename the copies automatically.
Hi Karl
I thought I had got this sussed but I cannot see what I am doing wrong. I had to add in some extra valiations and adapted the script as follows:
// allow either 999999 or 99A9999A or AA999999 or 9999999
var re1 = /^\d{2}[A-Z]\d{4}[A-Z]$/;
var re2 = /^\d{6}$/;
var re3 = /^\[A-Z]{2}\d{6}$/;
var re4 = /^\d{7}$/;
event.rc = true;
if (event.value != “” && !re1.test(event.value) && !re2.test(event.value) && !re3.test(event.value) && !re4.test(event.value))
{
app.alert(“The entered value needs to be in the format of ‘999999’, ’99A9999A’, ‘AA999999’ or ‘9999999’.”);
event.rc = false;
}
The third one will not work. I also tried doing it as
var re3 = /^\[A-Z][A-Z]\d{6}$/;
and this does not work either.
I am confused as everything I have read tells me this is correct. Can you advise where I am going wrong.
Many thanks in advance.
Terry
Terry, you have a ‘\’ between ‘^’ and ‘[‘ – I am pretty sure that that’s the reason why our expression is not working.
Mike, I am sorry, but I don’t understand what your question is. What are you trying to accomplish?
Bobby, to validate your whole form, you need to run a loop over all fields that are required and then only run the second part of your script if the first one does not report a problem. Take a look here for an example: https://answers.acrobatusers.com/How-require-fields-filled-mailDoc-q140100.aspx
For a “trusted” function, you would need to install a script on every computer that could potentially submit your form, which in most cases is not something you can do. Here isa tutorial about using trusted functions: https://acrobatusers.com/tutorials/using_trusted_functions
Many thanks Mike, a second pair of eyes picks it straight away. Works perfectly now.
Terry
Terry, I don’t know who Mike is, but I’ll still take the credit 🙂
Hi Karl!
I have a field date named (TXT_DATE) which has the following format: yyyy-mm-dd. When a user click in the field, it prints the current date. Great! However, I would like to display a warning message when the user write an invalid date format. I require your help, please. Thanks in advance
bis dat, qui cito dat
thanks for support
Mike
Maud, the easiest way to accomplish this is by setting the field’s format information so that it only accepts dates: Open up the field properties dialog, then go to the “Format” tab and select the “Format category” as “Date”. You can then either pick from a number of pre-defined formats (e.g. “yyyy-mm-dd”), or define your own format by selecting “Custom”. Now Acrobat will actually make sure that the information entered is a valid date.
i wanted a java script i can use to validate email address on the form i am creating with adobe form creator.
thanks
Newish, this is actually nothing specific to Acrobat’s JavaScript and you can find numerous samples on the Internet when you search for “Javascript validate email address”. Here is an old stack overflow question with a few good answers: http://stackoverflow.com/questions/46155/validate-email-address-in-javascript
Hi Karl !
Thank you for the previous support. I have a numeric field with nine characters max. I would like it to start by the figure 1. I require your help please.
Thanks
Karl,
I’m new to Js . Your information has been very helpful. From your sample script I tried to insert validation code to check to see if the field the customer entered has at least 1 uppercase alpha character, at least 1 lower case alpha character, include at least 1 number or special character, and be a minimum of 8 characters. My code is below:
event.rc = true;
if (event.value != /^(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[^a-zA-Z0-9])(?!.*\s).{8,15}$/)
{
app.alert(“The password requested must have at least 1 uppercase and lowercase alpha character, at least 1 number or a special character!”);
event.target.textColor = color.red;
}
else
{
event.target.textColor = color.black;
}
Creating a form that needs an option that if you click a check box, a second Print Name & Title line and a Second Signature line would show up (if check box is not checked, then the second Print Name & Title Line and Second Signature line are hidden). Does anyone have a code, script or instructions on how to get this done?
I’m fairly new to this and need to get the form completed ASAP.
Andrea, you can create the fields and then hide them, and only show them when they are needed. Use the Field.display property for that: http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_API_AcroJS%2FField_properties.htm%23TOC_displaybc-19&rhtocid=_6_1_8_31_1_18
Jack, you may want to review how regular expressions work in JavaScript. Take a look here for example for some information about how to approach this: http://www.the-art-of-web.com/javascript/validate-password/ (keep in mind that you cannot just use web browser JavaScript in Acrobat, you will need to adjust the programs). In general, to test if a regular expression is true you would use something like this:
if (/[A-Z][a-z][0-9]/.test(event.value)) {
// do something
}
Hi Karl!
I don’t know anything about JS so your help would be very appreciate Karl.
I want the excel to integrate with my acrobat.
When I input text in a blank field, it will automatically find and detect text if there’s any information available in the excel file then it will show into another blank field. If not any information there, it will show none.
Something like these;
Client Name: AXY
Instruction: AXY sample text (if any information available in excel)
Instruction: NONE (if any information is not available in excel)
Is that possible?
Jonathan, no, this is not possible, at least not without a lot of other “stuff” that needs to happen outside of the PDF form. There is one way you can accomplish this, but that requires different software, and a different type of form: Adobe actually has two different competing form systems in PDF documents: AcroForms, which are the forms you can create in Adobe Acrobat, and XFA or LiveCycle Designer forms, for which you need the LiveCycle Designer application. With Designer, it’s possible to use a form that connects to a data source on Windows. That data source can be an Excel document. This will work as long as you are using Adobe Acrobat to fill out the form, but will fail if you only have the free Reader. And, it’s a lot more complicated to setup such a form. There is one method you can accomplish something like this, but the data would not come directly from the Excel document: If you can export the Excel document to e.g. a tab separated values text file, or a CSV file, you can embed that in your PDF form, and then the form can take the data from that embedded document to fill in these other form fields. If this is something you would consider, feel free to get in touch with me via email for my consulting services.
Dear Sir.
I want JavaScript use for PDF form Fillable. When i can Input Data QUANTITY & PRICE Filed Then Show Data in AMOUNT Field . Other wise AMOUNT filed always Hidden. How can solve this problem Script?
I want like best cooperation.
|Sabir Rana
Sabir, take a look here for a tutorial about how to show/hide form fields based on different conditions: https://acrobatusers.com/tutorials/show_hide_fields
Hi all,
I’m very new to PDF Form and the scripts, hoping someone could help me out.
I needed PDF Form to be able to:
Auto-populate Field 1 as “K” if Field 2 has a “value”
Auto-populate Field 1 as “P” if Field 3 has a “value”
Please advise.
Thanks in advance!
Hi!
I need to update a date field on a fillable Adobe form. It will let me display it as July 25, 2017 but I need additional days displayed. I need it to show July 25-27, 2017. Even when I try custom, I cannot input it to show up this way. The closest thing is mmmm d, yyyy. Please help!!!
Thanks, Jamie
Jamie, there is no standard field that would allow you to specify a range of dates. You will have to implement that on your own using JavaScript.
Winston, something like this should work for your “Field 1” – use this as the calculation script for the field. However, the problem is what should the script do when both fields 2 and 3 have a value. In the following script, one value will override the other.
event.value = "";
if (this.getField("Field 2").value != "") {
event.value = "K";
}
if (this.getField("Field 3").value != "") {
event.value = "P";
}
Hi!
I would like to put a restriction on the PDF form so that if neither of the values are entered the Per(Km) field/column would be left blank . In this manner the Total filed i.e.[distance * Per(Km)] wouldn’t auto-calculate. Could you kindly suggest an extension/addition to your code.
The code I used based on your valuable inputs is mentioned below for your kind perusal:
——————
event.rc = true;
if (event.value != “” && event.value != “3.5” && event.value != “7”)
{
app.alert(“The entered value needs to be either ‘3.5’ or ‘7’!”);
event.target.textColor = color.red;
}
else
{
event.target.textColor = color.black;
}
—————–
Hi,
If i want to prepare such a form in pdf if the first field of the form is not properly filled then it will allow to move on the second field and like wise.
If such validation is possible in pdf please help me to create that.
Regards,
Saurajit Chaudhuri
Saurajit, yes, this can be done, but from a usability perspective, it’s not a good idea. It’s much better to keep the field marked as “needs to still be filled out”, and then at the end, provide information to the user about which fields are still blank. If you do want to go forward with this, I can certainly offer my professional services. My contact information is on the “About” page.
Aristotle, you can set the field to blank, by adding the following line to your if condition:
event.value = “”;
I need to send one of two fields from my software to a PDF document. It will be either or but not both fields. For example, the user could select one of two types of identification used to identify a customer. They enter the ID type and information in two different fields depending on whether the ID used is a state issued ID or some other form of ID.
I’m filling the document with text fields fed directly from my software.
How do I do this?
I have a question on calculating a discount based on a date.
Inside the form there is a 20% discount if a product is ordered prior to a specific date.
The quantity is multiplied by the cost and the discount is applied if it is prior to a specific date.
I could use some help as to how to incorporate that.
Greg, what you want to to does not require a custom validation script, you need a custom calculation script. Similar but different. You would add a “Discount” field that would use a custom calculation script. This script would get the date, compare it to the cutoff date for the discount, and if it is before, calculate the discount as 0.2*quantity*cost – if the date is later than the cutoff date, the discount would be set to 0. When you then calculate your total, you would sum up all sub-totals (in case there are more than one), and subtract the discount (either a calculated value, or 0, based on the logic in your custom calculation script).
Brenda, this is not something you would do with a validation script, it’s done in a calculation script. The general outline would be like this:
var theType = this.getField("TheType").value;
var theValue = "";
if (theType == "StateID") {
theValue = this.getField("Field_1").value;
}
else if (theType == "OtherID") {
theValue = this.getField("Field_2").value;
}
else {
theValue = "";
}
event.value = theValue;
Karl, I was hoping you could advise me how to validate radio buttons? Does the custom validation script go in the button themselves or in my custom submit button? Any help would be great.
Stephanie, radio buttons and checkboxes do not have a validation function. There are other was to validate a setting in a radio button group. You can for example use a calculation script of an otherwise hidden and read-only text field. Whenever the radio button state changes, that calculation script will get called, and you can validate your radio button settings. You an also defer the validation until the user clicks on the submit button, but it might be more straight forward to display the error message right away when the user actually performs the operation that results in the validation error.
Karl, So if you were to use the example you gave for a hidden read=only text field how would you validate this? sorry I too like most of these users are new to javascript.
Stephanie, there is no one size fits all script for this. I don’t know what our requirements are. Let’s say you have a field named Field1, and if there is any information entered in that field, you cannot use Choice3 in your radio button group. The following should work:
// validate the radio button group "Group1" based on the field "Field1":
var fieldContent = this.getField("Field1").value;
var buttonInfo = this.getField("Group1").value;
if (fieldContent != "" && buttonInfo == "Choice3") {
this.resetForm("Group1");
app.alert("'Choice3' is not a valid selection");
}
All my radio buttons are yes or no. besides one that has you choose 1st, 2nd, or 3rd shift. I just want them to be required to fill out and not be blank prior to submitting/emailing the pdf.
hi sir ,
i have problem. i have form where two date field available, when date is enter between 2/23/2006 to to 2/30/2007 in date box then show john is CEO in text field.if we enter date 2/24/2007 to 2/30/2008 then show amir is admin in same text field.please help me sir.
shoaib, you would do this with a custom calculation script that tests if the data range is between the first set of dates, or the second set of dates, and then selects the appropriate value for your text field. However, your two date ranges are overlapping, which means that you would also need to come up with a strategy to deal with that overlap.
I have 2 date fields on my PDF form. Test Date and Live Date. I need a validation for Test Date to be >=Live Date
Hi!
I need a update in date field on a fillable Adobe form. I can able to auto fill the date(when ever the Form is opened) in date field if the form is in English language, I need is, if the form is in other language (ex: Spanish), I need the auto filled dated should display in Spanish (Ex: 19 Enero 2018)
Please help!!!
Thanks, Madhi
Madhi, unfortunately, there is no easy way to do this. Acrobat’s Date object does not support the toLocaleDateString() method, so you need to implement this on your own.
Karina, you will first have to parse the date strings from the fields and convert them to date objects, then you can compare the dates directly. Something like this should work as the validation script for the second field:
var thisDate = util.scand("mm/dd/yyyy", event.value);
var thatDate = util.scand("mm/dd/yyyy", this.getField("Date1").value);
if (thisDate < thatDate) { app.alert("Error"); } event.rc = (thisDate >= thatDate);
Can you help with some code.
table with 6 columns, I would like an alert in the to pop up if the total exceeds $1500 (without clicking into the total column).
Ta.
I am trying to get a form to validate with a percentage that if it goes over a certain percentage then the text will turn to red. I either need it to do it thru validation but without the popup warning or thru the calculation. This is currently what i’m using for the calculation of the script
(function () {
// Get the field values, as numbers
var v3 = +getField(“payment”).value;
var v4 = +getField(“ApplicantsTotalNetIncome”).value;
var v = v3 / v4;
if (!isNaN(v) && v4 != 0) {
event.value = v;
} else {
event.value = “”;
}
})();
For validation i’m using
event.rc = true;
if (event.value != “” && event.value != “0.00” && event.value != “0.30”)
{
app.alert(“The entered value needs to be either ‘0.00’ or ‘0.30’!”);
event.target.textColor = color.red;
}
else
{
event.target.textColor = color.black;
}
The problem with the validation is that it’s giving me a popup and will only allow me to use 0.00 or 0.30 when i need it to go in between those and then if it goes over just to turn to red. i don’t need the popup warning.
Advise would be really helpful..
Tom, is the data in your table in PDF form fields? If so, you would use a custom calculation script to do that. In this calculation script, you can sum up the individual fields, and then compare the outcome of that calculation to the value 1500 and if that is greater, use the “app.alert()” method to display a message.
hmm it’s saying my comment is waiting moderation
Zachary, the behavior you see is not surprising, that’s what you’ve programmed. You need to understand the comparison operators in JavaScript to change your code. You say you want all values to be acceptable, but turn the field content red when it’s outside the range of 0 <= x <= 0.3. To get rid of the popup, just remove the line that starts with "app.alert". You also need to change the first condition from an "and" to an "or". Try this:
event.rc = true;
if (event.value < 0.00 || event.value > 0.30) {
event.target.textColor = color.red;
} else {
event.target.textColor = color.black;
}
Zachary, it was waiting in moderation because my paying customers always get priority, that means that sometimes you have to wait a bit longer for free help.
Hello Karl,
Thanks for the reply, how would I code if greater than – then Appalert? Can you assist.
Thank you, I actually got it to work the day before you responded lol. I ended up using this code and it seems to work the way i need it to
(function () {
// Get the field values, as numbers
var v3 = +getField(“applot”).value;
var v4 = +getField(“coapptotnet”).value;
var v = v3 / v4;
if (!isNaN(v) && v4 != 0) {
event.value = v;
} else {
event.value = “”;
}
event.target.textColor = event.value <.30? color.black : color.red;
})();
the problem I am having now thou i'm not sure what to do. I am assuming this might be do to the tab order but i'm not sure. I have created a document using pretty much all Adobe for creation besides a few things that were transferred from a word document. I created the form and have all the for field working like they are suppose to but now when i try to click on certain fields it's wanting to select text in the background rather then letting you click on the form field that needs to be filed out. Its acting like layers to me like what i would see in photoshop. is there any way that you know to get it where the only thing that can be selected is just the form fields that need to be filled out and not selecting any of the text in the background. Thank you and sorry if it seemed like i was being disrespectful not use to having to come to someone for help on stuff. usually i figure it out on my own but i have been stuck on this one now for around a week and cannot seem to find a solution with all my searching online.
Hi Karl,
I’m pretty much a novice and need some assistance:
I have a drop down menu with several choices (let’s say A, B, C, D, E) and I have a mutually exclusive Yes-No check-box that is required. I have it set to always default to No, but I want to have it automatically switch to Yes when Option E is selected in the drop down.
Any direction will be appreciated.
Thank you!
Hi I would like to ask how to do validate and print in PDF forms
Hi,
I need to dynamically add three fields when a button is clicked. The fields I need to add are equipment type, make, and model. Each time the button is clicked I need all three of these fields to pop up. I might have 1-7 different types of equipment on the job and need to be able to add these fields if I have multiple pieces of equipment. Is this possible?
Hi, I need help writing a validation script to create a dependent drop down list.
This is an example of what I need:
Box A has numerous options.
If Box A is filled in with “x”, then Box B will display results “1,2,3,4,5”.
If Box A is filled in with “y”, then Box B will display results “6,7,8,9,10”.
If Box A is filled in with “z”, then Box B will display results “11,12,13,14,15”
And so forth.
Thanks for your help!
I need to design this code that will show me the amount calculated automatically and if there is any coupon code that is used by my customer so discount is cut off automatically and I am shown the whole detail.
La Cusioun, what you want to do has nothing to do with validation scripts. You need a custom calculation script for that. Here is some information about how to do calculations in a PDF form: https://acrobatusers.com/tutorials/print/how-to-do-not-so-simple-form-calculations
Good Afternoon:
I’m hoping you can help me. I only have a small understanding of Java Script. I am creating an Acrobat Pro DC form that has drop down lists and I have a default value that I want something other than the default value to be selected. I have made the field required. So the form looks something like this . . .
Field Name: ProposalType
Dropdown List:
Proposal Type – Select One <<Default Value
Professor
Associate Professor
Assistant Professor
I want an error message to show up if the end user tries to click out of the drop down box without selecting an option other than the default value.
I found this JavaScript but do not know how to make it work. Can you help?
if (event.value != event.defaultValue) {
// do something;
app.alert("Required field not completed.\nField name: " + event.target.name, 1, 0);
}
Hi Karl,
I’ve got a form field that checks another field’s value to see if the data populated has to do with a ‘client service’ member – and if so, it displays the text as a % formatted string, else it defaults to a $ formatted string. It’s ok if they’re shown as text strings – as the values in those fields are not being used in any calcs anywhere else…. but one thing I’d like to do is, that if the enteref value is a $ value – to use parentheses and red text to show a negative currency value… I have the following script that does the % vs. $ check, but haven’t been able to modify it to get the color and parentheses to show up when I’m showing a $ value…
i.e. if the template is ‘client service’ show the value of the field as a %, otherwise, show black currency $N,NNN.N if positive, or ($N,NNN.N) in red if negative…
var Template = getField(“Template”).value;
if (Template ==”Client Service”){
event.value = util.printf(“%.1f%”,event.value*100);
}else{
event.value = util.printf (“$%.1f”, event.value);
}
Not sure if I it’s best to do one part of this in the custom format script part, and a validation script, or all in one place… right now the above script is a validation script.
Using the script originally posted at the top of this thread, I’ve been trying to format a form field to only allow numbers and/or a % sign to be entered into a field.
event.rc = true;
if (event.value != “” && event.value != “AAAA” && event.value != “BBBB”)
{
app.alert(“The entered value needs to be either ‘AAAA’ or ‘BBBB’!”);
event.rc = false;
}
I can’t quite figure out what to change the AAAA and BBBB to in order to allow only numbers, in any string length, and/or a % sign to be entered into the form field.
Hello, this is a really useful site. I see people doing something similar to what I need to do. I am trying to add a 3% charge for someone that pays by Credit Card. My thought would be if they put information in the field CC# then it would add in the 3%. If not then it doesn’t add it in. I am open to an option where I have a radio button that says cash, check, money order, or credit card. If they select credit card then they would have to fill out the CVV, the CC#, and expiration date fields.
Karl,
Is there a custom validation script that will allow people using my fillable PDF form to import an image from their personal drive simply by selecting a pre-configured button?
RO1 Tech – this would not be done via a validation script, but through an action on a button. Any recent version of Acrobat or Reader can do this by just adding an image field to your form, you can can also do it the old fashioned way. See here for how it’s done (this post is about the feature that is built into Acrobat, but also lists the one line script): http://khkonsulting.com/2017/01/new-form-field-types-in-acrobat-dc-image-field-and-date-picker/
I have more than 20 checkboxes and I want when i check one checkbox other checkbox should automatically get uncheck. At a time user should only be allowed to check one checkbox.
Please help
Prachi, the easiest way to accomplish this is by creating a “checkbox group”, where all checkboxes share the same name, but have different export values. You will get the desired behavior automatically.
Hi Karl
I am having trouble with getting the test for text color to validate – I have tried your instructions at
“Tom, the following script will loop over all fields and check for the textColor == color.red condition:”
and even dumped the textColor for each field into the console – but despite some fields being marked in red, the console shows only G, 0 for all the fields.
I have checked the properties for the fields in question, and it definitely shows red.
Do you have any ideas on what is going on? I am using Nitro so it is possible that it is a bug specific to this program.
Unfortunately there is very little information available so I’m really hoping you can shed light on this issue.
thanks in advance,
Simon
Hi Karl
I found a solution to my validate based on color – the problem seems to be a bug in Nitro – so textColor wasn’t recognised, but fillColor was.
so for highlighting errors I ended up using
event.target.fillColor = color.red;
and for validation
if (f1.fillColor == “RGB,1,0,0”)
which now works fine.
Just putting it out there in case anyone else is having this problem
I’m not trying to do anything fancy (lol) just a simple script and mask. Currently, in the custome keystroke script I have:
if(!event.willCommit)
event.change = event.change.toUpperCase();
and that is working just fine to make the data in the field to always be uppercase, but since I cannot use an arbitrary mask and custom options at the same time, I decided to try and write a custom format script. this is what I have:
event.rc = true;
if (event.value != “” && event.value != “AAAA-9999”)
{
app.alert(“The entered value needs to be ‘AAAA-9999′”);
event.rc = false;
}
Whenever I type a value in (ex. MECH-4732) it will give me the error (alert that I wrote) that the value needs to be AAAA-9999. Maybe I misunderstand how to use the script.
Immediate help needed. Thank you
Roseline, the error message is pretty clear: You can only enter “AAAA-9999” – anything else will get rejected. This is not a format string, this is a literal string constant. To get the behavior you want, you need to use a regular expression. Something like this should work:
event.rc = true;
var r = /^[A-Z]{4}-\d{4}$/g;
if (event.value != "" && !r.test(event.value))
{
app.alert("The entered value needs to be in the form of ‘AAAA-9999'");
event.rc = false;
}
The AAAA or BBBB example was perfect for my purposes!
Hi Karl. I stumbled upon this thread looking for information on javascript validations. I’m using the the script:
var n = event.value.indexOf(“leadership”);
if (n == -1) { // substring not found
app.alert(“Does not contain one of DOR’s Core Values.”);
event.rc = false;
}
else {
event.rc = true;
}
to require certain words in a field box. However, I need more than one word to be required. Such as it must contain “Teamwork” OR “integrity” OR “respect”. Not all three of the words. How can I change the script so it doesn’t require all of the words, but at least one of the words?
Thank you
Rhnea, there are different ways you can do that. One option is to change the test for the if structure from “if (n==-1)” to this:
if (event.value.indexOf(“Teamwork”) == -1 && event.value.indexOf(“integrety”) == -1 && event.value.indexOf(“respect”) == -1)
In this case, if the string does not contain “Teamwork” and does not contain “integrity” and does not contain “respect”, the error is being displayed. You may want to do a case independent check (unless you can guarantee that the spelling is always the same). To do that, you can convert the string you receive to e.g. lowercase and then compare with the lowercase version of your target string:
event.value.indexOf(“leadership”).toLowerCase() == "teamwork"
Hi Karl,
I just stumbled on your site this morning. Very impressive and useful.
I have a situation that falls into the validation area but involves a checkbox.
I have a form that provides for four states, the first of which is adressed by a check mark the rest are text entries.
My first solution is to use two fields: a check box and a dropdown with the three text entries.
As they are mutually exclusive and dependent on the state of the checkbox, I created the following script for the checkbox in the actions section…
var f1 = this.getField(‘cbNoLoadTest01’);
var f2 = this.getField(‘Dropdown3′);
if(f1.value ==’Off’){
f2.display = display.visible;
f2.value = ‘N/A’;
}
else
{ f2.display = display.hidden;}
I am intrigued by your answer to Hugo in December 2015, where you advise putting the following code in the text validation for the initiating text field…
var f = this.getField(“Rationale”);
if (event.value == “”) {
f.display = display.hidden;
}
else {
f.display = display.visible;
}
The concept seems similar to what I am using and I am wondering if there is another way to get the action that I want and retain the checkmark that the client wants.
I like the use of the variables for the field names as the form contains a large number of the field pair which makes repetition easier.
Is there a way to make these two controls a group?
I am getting used to this form of Javascript and would like to know what is the latest version of the SDK… a link would be greatly appreciated as well as your suggestion(s).
Thanks
Hi Karl,
In the form that I referenced in my previous message, I notice that all the checkboxes appear as checked when the form opens; even though I turned them off in properties.
Can I run a general script on open to select ALL checkboxes and set them to off?
My toggle script for checkboxes is an action and works on mouse up
I hope you can help me. I have a pdf where the user types the “BeginningDate” and “EndingDate” ( those are the field names).
Then as they enter bank transactions, they will type each transaction date in the form below. I am needing a script to validate the date typed for each transaction is within the “BeginningDate” and “EndingDate” typed at the top.
I’m getting a NaN, on a field that uses the following javascript. I can’t figure out why or how to fix it. The calculation does work. Any help would be appreciated.
Regards,
jer
/************ add global script for calculation *******************/
var hdCnt = +0;
var hdBkInNbr = +””;
var hdCaseNbr = +””;
var tltCredit = +0;
for ( var ii = 1; ii < 31; ii ++)
{
var bookIn = this.getField("BookIn " + ii).valueAsString.toUpperCase();
var caseNo = this.getField("CaseNo" + ii).valueAsString.toUpperCase();
var credit = parseInt(this.getField("BT Days Credit" + ii).value);
if (ii == 1)
{
hdCnt = ii;
hdBkInNbr = bookIn;
hdCaseNbr = caseNo;
tltCredit = credit;
continue;
}
//if (bookIn == "" || bookIn == hdBkInNbr)
//{
if (caseNo == "" || caseNo == hdCaseNbr)
{
tltCredit += credit;
continue;
}
//}
this.getField("Total BT Credit" + hdCnt).value = tltCredit;
this.getField("Total BT Credit" + ii).value = credit;
// reset values
hdCnt = ii;
hdBkInNbr = bookIn;
hdCaseNbr = caseNo;
tltCredit = credit;
}
/************ end *******************/
jeramirez, without knowing more about your PDF form it’s impossible to say what’s wrong. You said that your code is working, so I assume the problem is wth the field. The first think I would check is to see if the field type is incompatible with the output your script produces. To do that, change the text field to just plain text (remove any formatting or validation rules). Does it work? If so, then look closely at the output that is generated.
Bryan, what you want to do is plain old JavaScript and does not have anything to do with Acrobat. Look at the “Date” object and then do a comparison (or actually, two because you want to check that the date is within a range).
Ken, yes, any document level script will run when the document is opened.
Tom, the latest version of the SDK is always in sync with the latest version of Acrobat (or lagging slightly behind when a new version comes out). However, JavaScript does usually not change from version to version, so any SDK version that is recent (e.g. any DC version) is sufficient. So whatever you have will work. I use this link for JavaScript documentation: http://help.adobe.com/en_US/acrobat/acrobat_dc_sdk/2015/HTMLHelp/index.html#t=Acro12_MasterBook%2FJS_Dev_Overview%2FJS_Dev_Overview.htm
As far as the checkbox problem goes, add the script to the mouse down event hander for the checkbox.
I have a created a form wherein there are two fields Field#1: Job Title with Dropdown list:
Software Developer
Systems Analyst
Systems Admin
and Field#2: Job Duties.
I want to have the Job Duties in Field#2 based on the field#1 value. How do I get that?
Syed, take a look here for information about how to create conditional dropdown controls: https://answers.acrobatusers.com/Conditional-Drop-Down-Help-q287268.aspx
Hi Karl,
We are looking to provide a validation script for a form field which if not completed (and tabbed away from) will come up with a message saying “This field is required”. We can get the message to appear when you enter text in the field, delete the text and then tab out of the field, however, the message won’t come up automatically when there is nothing entered into the field and you tab away from it. Any suggestions?
Thanks so much!
Corinne,
use the “On Blur” field event to check when the user leaves the field. This way, even the empty string can be flagged.
Thanks Karl Heinz Kremer for your post dated: November 6, 2014 at 3:38 pm using this statement wanted to have popup msg for text field to enter only characters and it worked fine.
Thanks again…
Hi all, I would love some help, within the format category under special there is a option for phone number but the amount of digits are limited, i’m from the UK and need to be able to input 12 digits. I can achieve this in the Arbitrary Mask, but I like the way that when selecting this field on a smart phone or tablet the Numeric keypad is forced so although the Arbitrary mask works it does not force the Numeric Keypad. Is there a salutation to be able to enter a 12 digit number and forcing the numeric keypad when filling in the form on a smart phone or tablet? thank you in advance..
Wayne, I am not aware of anything that would do that.
total newbie here
i have a field that must be divisible by 25
0 is acceptable or blank is acceptable
how would i code that?
Is there a scripting way to read values from PDF form fields from all PDF’s in a particular folder, and then store those values as a CSV file in the same folder. Aka: ‘Merge data files into Spreadsheet’ option, but via a single script?
Pam, you may be able to do this with a JavaScript action that processes all files in a folder. You would however have to get the field content for each field and store the content on a per file basis in an unrelated file that you either create or if it exists, append to. The combined data would then have to be extracted from that file. I would however approach this differently and write a Visual Basic program that opens the folder and then uses Acrobat’s IAC interface to process each file. To get the field contents, you can use the mechanism I describe here: http://khkonsulting.com/2010/09/reading-pdf-form-fields-with-vba/
Isabelle, you would need a validation script that checks the remainder of a division. The % operator will do that:
event.rc = (event.value % 25) == 0;